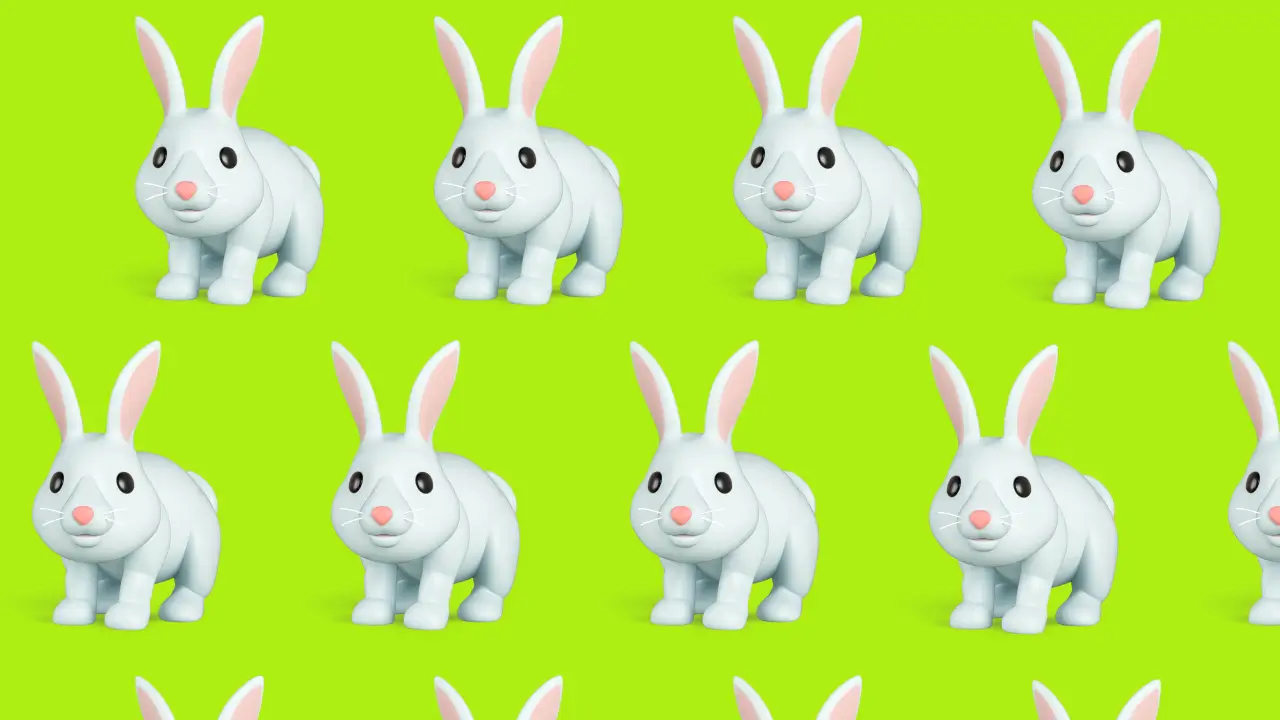
Startups
Small Business Odyssey: Navigating the 2024 Landscape5 trends for small businesses
December 26, 2023Enhancing UX and performance with debounce techniques
December 26, 2023
By: Maria
Imagine you’re typing a query into a search bar, and with every keystroke, there’s a delay or a lag – frustrating, right? This is where JavaScript debouncing comes into play, a technique as essential as a good cup of coffee for developers looking to enhance user experience and optimize app performance.
Picture this: you’re creating a snazzy new app. It’s sleek, it’s sexy, but oh boy, does it have a ton of events! Clicks, scrolls, you name it. Each of these events acts as a tiny taskmaster, demanding attention from your app. Too many of them, and your app’s like a juggler with one too many balls in the air – bound to drop one (or all) eventually.
Debouncing is like that cool, calm friend who knows when to take a step back. It’s a technique where we say, “Wait a moment before we do this again.” In technical terms, it’s executing a function or event only after a specified delay has passed since its last execution.
Let’s dive into the nuts and bolts of how debouncing works in JavaScript.
This function receives any type of callback function and the time in milliseconds when the callback function should be executed.
function debounce(callback, wait) {
let timeout;
return function executedFunction(...args) {
const later = () => {
clearTimeout(timeout);
callback(...args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
}
This callback function is responsible for getting the string value from a search input. The user types what they want to search, and it’s received here. This is also where you could make a request to an external API to query a database.
function handleSearch(e) {
console.log("searching for: ", e.target.value);
Using both functions
const debouncedSearch = debounce(handleSearch, 500);
Adding the event to the input in question so that each time the user types a letter, the callback is triggered.
document
.querySelector("#search-input")
.addEventListener("input", debouncedSearch);
This snippet of code is your golden ticket. It creates a function that limits how often a callback is executed.
Consider a search function in an eCommerce app. Without debouncing, every keystroke sends a request to the server – a surefire way to slow things down. By implementing debouncing, you limit requests, say every half-second, making your app smarter and more efficient.
Debouncing isn’t just for search bars. It’s a versatile tool in your coding arsenal. Use it for scroll events, window resizing, or anything where you need to control the rate of event firing. It’s about making your app not just functional but also smart and considerate of server load and user experience.
In conclusion, debouncing might not be the flashiest tool in JavaScript, but it’s certainly one of the most impactful. It’s about smoothing out the rough edges in your app’s performance, making sure that each user interaction is as seamless as it can be. Remember, in the world of app development, sometimes the best moves are the ones you don’t see.