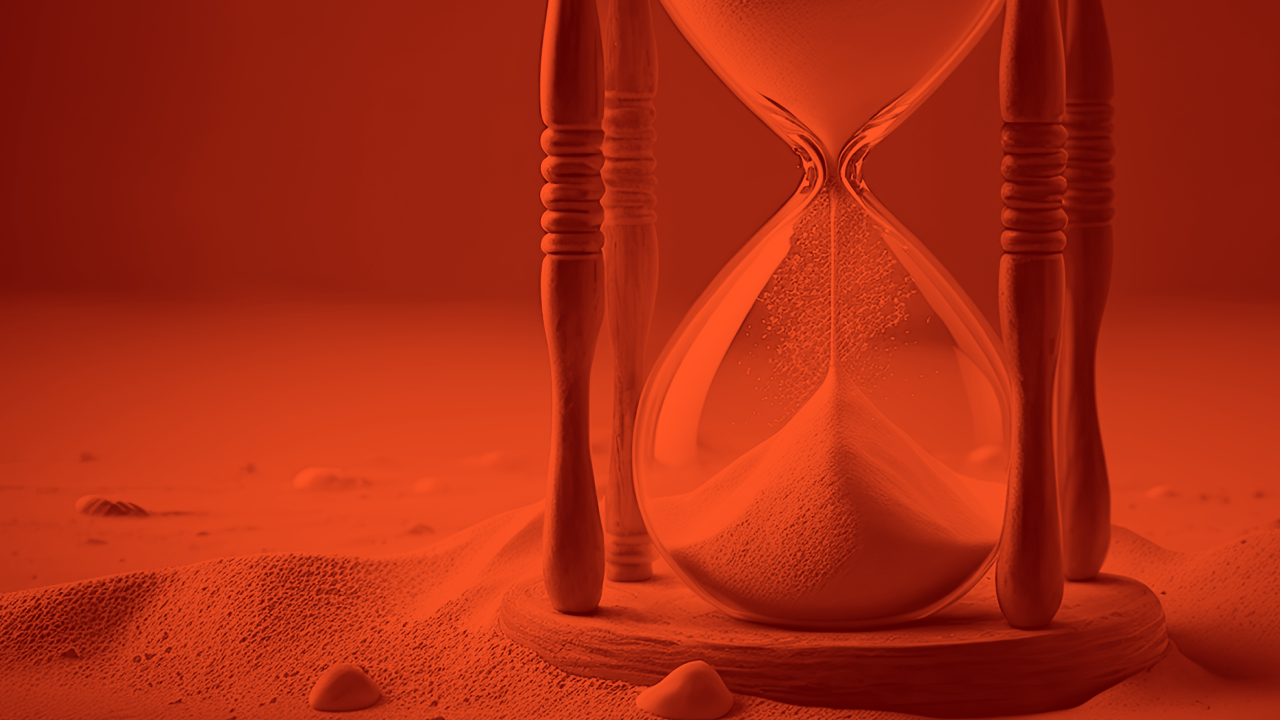
Works
Seize the Day Now: a Visit to the Carpe Tempus Product PageDeveloping the App and Website Together
November 28, 2023Enhance your code efficiency with a simple yet powerful tool
November 28, 2023
By: Heberlyn
As developers, we’re always on the lookout for efficient ways to streamline our coding process. Today, let’s dive into a JavaScript function that’s a game-changer: closest()
. This function is a hidden gem in the JavaScript toolbox, offering a straightforward solution to a common development challenge.
Imagine you’re working with a nested structure in your HTML, and you need to access a parent element with specific characteristics. Traditionally, you might find yourself cascading through multiple parent()
calls, which not only looks messy but can also be a headache to maintain.
closest()
is a function in JavaScript that I use to find the nearest ancestor of an element that matches a specific selector. Think of it as a shortcut through the DOM tree, getting you directly to the element you need. Here’s how I use it:
<div class="demo container">
<div class="demo"> Grandparent
<div class="demo">Parent
<div id="myElement" class="demo">The parent of this div element will be selected.</div>
</div>
</div>
</div>
In this example, closest()
efficiently locates the nearest parent element with the class โcontainerโ to “myElement,” avoiding the need for multiple .parent()
calls.
const element = document.getElementById("myElement");
const closest = element.closest(".container");
if (closest) {
closest.style.border = "4px solid black";
}
This approach is incredibly efficient. You’re not just saving lines of code; you’re making your code more readable and maintainable. Plus, closest()
immediately makes it clear what you’re trying to achieve, enhancing the collaborative development process.
closest()
has proven to be incredibly useful in various scenarios.The closest()
function is versatile, fitting into various development scenarios. Whether you’re working on dynamic form validations, managing UI elements, or dealing with complex DOM structures, closest()
can be your go-to solution for handling parent-child relationships in the DOM.
To get a deeper understanding of closest()
, check out these resources:
Embracing closest()
in your development toolkit is more than just learning a new JavaScript function. It’s about adopting a smarter, more efficient approach to DOM manipulation. So, I encourage you to give closest()
a try and see the difference it makes in your development workflow.
Stay tuned to our blog for more JavaScript tips and insights. Keep coding, and remember, sometimes the simplest functions can be the most powerful!