You’re getting started with JavaScript and, suddenly, you’re hit with two mysterious symbols: {}
and []
. Both are brackets, but they’re worlds apart in JavaScript!
Let’s break down what makes them different—and why it matters when you’re building with JS.
Declaring Arrays with Square Brackets ([])
First up, we have the trusty []
, a.k.a. square brackets. These are the array markers, and they’re here to help you list out items in a specific order.
- Purpose:
[]
creates an array, an ordered list where you can stash multiple items. - Use: Arrays are ideal when you need an indexed collection, like a list of numbers, strings, objects, or even other arrays.
- Access: In arrays, each item has a specific position, known as an index, starting at zero.
let avengers = ["Iron Man", "Captain America", "Thor", "Hulk"]; console.log(avengers[0]); // Outputs: "Iron Man" console.log(avengers[2]); // Outputs: "Thor"
Using Curly Braces ({}) for Objects in JavaScript
Now let’s talk about curly braces {}
. These are for creating objects, which let you define collections with named properties.
- Purpose:
{}
is for creating an object, which is a collection of key-value pairs. - Use: Objects are perfect for representing complex entities with various properties—think profiles, settings, or configurations.
- Access: Objects don’t have indices. Instead, they use keys (usually strings) to label and access values.
let ironMan = { name: "Tony Stark", alias: "Iron Man", abilities: ["Genius-level intellect", "Powered armor suit", "Expert tactician"], age: 48 }; console.log(ironMan.name); // Outputs: "Tony Stark" console.log(ironMan["alias"]); // Outputs: "Iron Man"
Key Differences
Here’s a quick breakdown of how these two differ:
- Access: Arrays rely on numeric indices, whereas objects use named keys.
- Structure: Arrays are ordered collections, while objects are unordered.
- Use Case: Arrays work well for lists where order matters. Objects are better for collections where you need named properties.
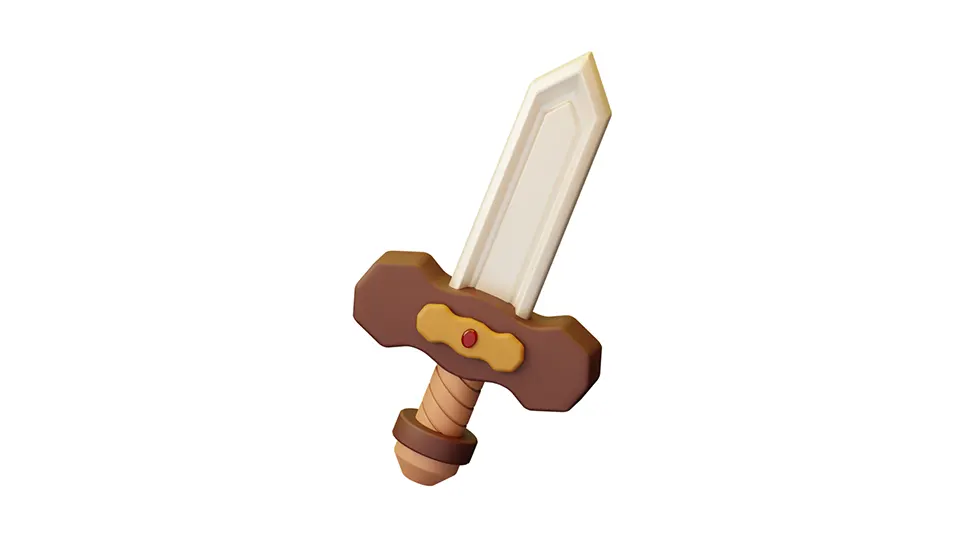
Advanced Differences Between Arrays and Objects
Let’s explore the technical side: JavaScript arrays aren’t like those in lower-level languages. They’re optimized for sequential access and work best with integer indices. Objects, on the other hand, are great for quick access with keys but aren’t ordered or optimized for sequential access.
1. Memory Layout and Performance Considerations
Arrays in JavaScript aren’t contiguous blocks of memory, like arrays in languages such as C or Java. They’re optimized for numeric indices, but they can store mixed types. Methods like .push()
and .pop()
are fast due to these optimizations.
Objects are essentially hash tables, making key-value access efficient but slightly slower than array access due to hash table lookup overhead. Numeric keys don’t provide any special optimization in objects, so arrays are generally preferred for numerical indices.
2. Use Cases and Performance Implications
Arrays are great for ordered lists where you need quick access by index, may include duplicates, and often utilize array-specific methods like .map()
, .filter()
, and .reduce()
.
Objects are ideal for key-value pairs with unique keys. Use them when you have sparse or irregular data, and when order isn’t important.
3. Alternatives to Arrays and Objects: Maps, Typed Arrays and Sets
For object-like collections with guaranteed insertion order and support for any key type, consider Map
. Maps are generally more efficient than regular objects for large numbers of dynamic keys.
const heroPowers = new Map(); heroPowers.set("Iron Man", "Powered armor suit"); heroPowers.set("Thor", "God of Thunder"); console.log(heroPowers.get("Iron Man")); // Outputs: "Powered armor suit"
If you need to handle large quantities of numerical data, TypedArray
(like Int32Array
, Float64Array
) provides fixed-length, typed buffers for faster processing, especially with binary data.
When you need an array-like structure without duplicates, Set
offers unique items and fast membership checks.
const uniqueAvengers = new Set(["Iron Man", "Thor", "Iron Man"]); console.log(uniqueAvengers.size); // Outputs: 2 (no duplicates)
WeakMap/WeakSet is great for scenarios where you want garbage collection of keys or values no longer referenced elsewhere in memory.
4. JavaScript Engine Optimizations
Arrays optimized for homogeneity (e.g., all numbers) perform better in most engines. Mixing types may de-optimize the array.
Objects with static, predictable properties are faster due to inline caching. Avoid adding or deleting properties dynamically to maintain performance, as this can disrupt engine optimizations like hidden classes.
5. Choosing the Right Data Structure
Array vs. TypedArray: TypedArray
is best for binary data handling and raw performance.
Object vs. Map: Map
shines with dynamic or non-string keys and high-performance needs.
Set: Ideal for collections where uniqueness matters and duplication is undesirable.
Now That You Know, Use Them as You Need
And there you have it! Use []
for ordered lists and {}
for complex entities with properties. These brackets aren’t just symbols—they’re essential tools for organizing and structuring data in JavaScript. So, choose wisely and code on!