Ready for a magical coding trick? It’s high time we simplify your code, and guess what? REST is here to our rescue! With this power-packed tool in JavaScript and TypeScript, transform those extensive arguments into a neat, single function. Pretty cool, right?
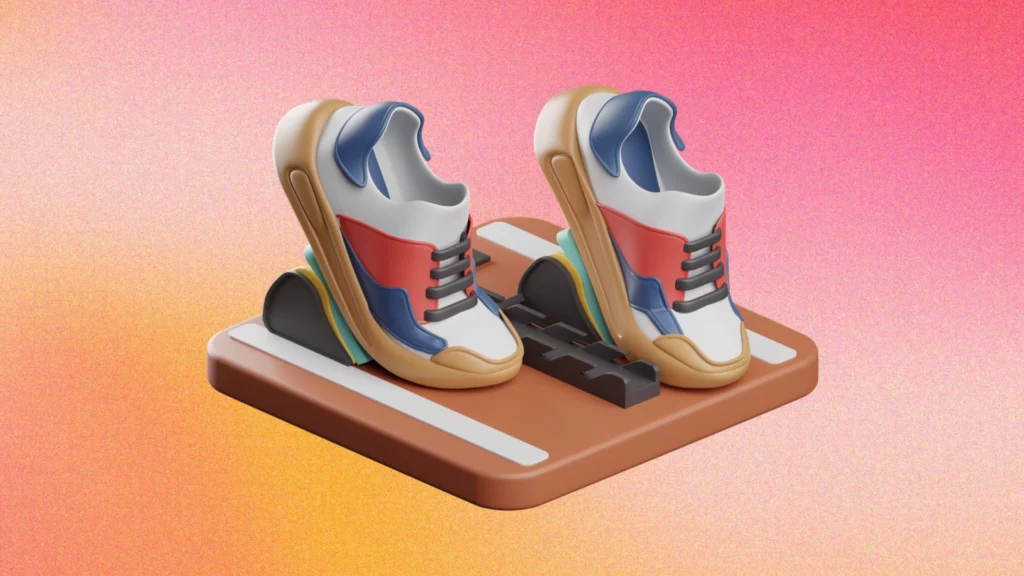
Shaping Up Your Array
REST, my friends, is not just a breather amidst a busy day, but a super handy parameter in Javascript and Typescript, known in the techie world as the spread syntax.
What’s the best part, you ask? It’s a piece of cake to pass any number of arguments to a function with REST! All you need to do is pass them as an array, and voila! You’re good to go.
Sounds too good to be true? Well, hold on to your hats, folks! REST not only simplifies your code but also makes it robust while being a treat for the eyes.
A One-Stop Solution!
Ever tried running functions in Node.js? Then you surely know the drill. You need to feed in a specific number of parameters to execute the functions without any glitches.
But, here’s the kicker: if you miss out on any crucial parameters, you might be staring at a broken code and potential errors. Now, who needs that, right?
Fear not! Our hero, REST, is here with its brilliant trick of using “…args”. With this, you can pass any number of parameters of any type – be it strings, objects, numbers – you name it! Your code runs smoothly, just like a well-oiled machine.
Let’s check out an example:
function sum(...args) {
let total = 0;
for (const arg of args) {
total += arg;
}
return total;
}
sum(1, 1); // 2
sum(1, 2, 3, 4, 5); // 15
sum(2, 4, 6, 8, 10, 1, 2, 3, 4, 5); //45
And just like that, your code gets a touch of simplicity!
Can’t get enough of our #bitstips? Broaden your understanding by visiting our related article on how to master a browser console!
✍🏽This article was written by our copywriter, Sofia, in collaboration with our DEV expert, Enrique.