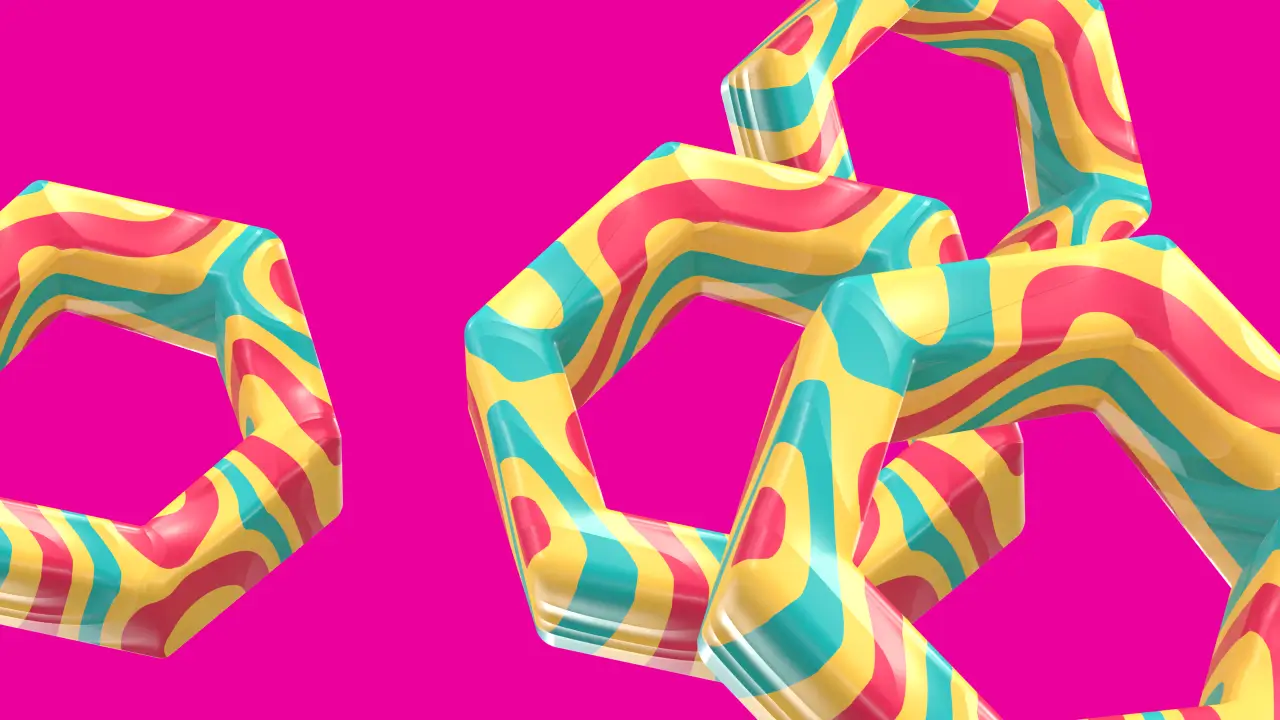
UX/UI
Back to the Future: Old School Meets New Cool in 2024 Web DesignTop trends for 2024 you can't miss
November 17, 2023Ready to build something extraordinary?
November 17, 2023
By: Michael Cardoza
Step into the forefront of web app development! Embrace the strength of Rust, a programming language revolutionizing web applications. Together, let’s unlock the power of Rust and its incredible capabilities.
Rust is celebrated for its safety and speed, revolutionizing web app development. Combined with Leptos, reminiscent of JavaScript frameworks like Solid, React, and Svelte, and Rust’s own Yew and Dioxus, Rust elevates its game. This duo makes Rust powerful and accessible, especially for those familiar with these frameworks.
Rust installation is your first step towards web development marvels, regardless of your operating system preference:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
rustc --version
and cargo --version
in a new terminal window.Trunk, a web assembly application bundler for Rust, is your next step. Install Trunk via Cargo and confirm its installation:
cargo install trunk
.
trunk --version
.Cargo streamlines the creation and management of your new Rust project:
cargo init awesome-web-app
. Cargo will create a new directory with this name and set up a basic Rust project structure inside.cd awesome-web-app
.src/
directory and Cargo.toml
Easily integrate Leptos into your project:
cargo add leptos --features=csr
. This command adds the Leptos crate to your project with the specified feature, “csr”.cargo update
: Upon adding the Leptos crate, Cargo automatically updates your cargo.toml
file with the new dependency entry. To compile code to WebAssembly for browser compatibility:
rustup target add wasm32-unknown-unknown
.rustup target list
.Start by creating a basic index.html
in your project’s root directory:
<!DOCTYPE html>
<html>
<head></head>
<body></body>
</html>
Add a “Hello, world!” message in main.rs
:
use leptos::*;
fn main() {
mount_to_body(|| view! { <p>"Hello, world!"</p> })
}
Launch and view your app at http://127.0.0.1:8080/ with trunk serve --open
.
Let’s spice things up with an interactive counter component.
Button
component in main.rs
that updates a counter when clicked.create_signal
function.
use leptos::*;
#[component]
fn Button() -> impl IntoView {
let (count, set_count) = create_signal(0);
let handle_counter = move |_| {
set_count.update(|n| *n += 2);
};
view! {
<div>
<h2>{move || count.get()}</h2>
<button on:click=handle_counter>"Click me!"</button>
</div>
}
}
The App component is composed of a header stating “Rust is Awesome” and an instance of the Button component.
...
#[component]
fn App() -> impl IntoView {
view! {
<h1>"Rust is Awesome"</h1>
<Button />
}
}
...
Here is the full code, if you want to copy it:
use leptos::*;
#[component]
fn Button() -> impl IntoView {
let (count, set_count) = create_signal(0);
let handle_counter = move |_| {
set_count.update(|n| *n += 2);
};
view! {
<div>
<h2>{move || count.get()}</h2>
<button on:click=handle_counter>"Click me!"</button>
</div>
}
}
#[component]
fn App() -> impl IntoView {
view! {
<h1>"Rust is Awesome"</h1>
<Button />
}
}
fn main() {
mount_to_body(App)
}
For that stylish look, add leptos_meta
as a dependency and create a CSS file with your desired styles. Then, integrate these styles into your Rust components to bring them to life!
cargo add leptos_meta --features=csr
Now, create a css file style/output.css
with this code:
body {
background-color: #0b0a11;
font-family: Arial, Helvetica, sans-serif;
}
main {
margin: 0 auto;
max-width: 600px;
text-align: center;
color: white;
}
.counter {
display: flex;
flex-direction: column;
justify-content: center;
}
.counter button {
height: 40px;
width: 120px;
border: 0;
margin: 0 auto;
}
Here is the full code:
use leptos::*;
use leptos_meta::*;
#[component]
fn Button() -> impl IntoView {
let (count, set_count) = create_signal(0);
let handle_counter = move |_| {
set_count.update(|n| *n += 2);
};
view! {
<div class="counter">
<h2>{move || count.get()}</h2>
<button on:click=handle_counter>"Click me!"</button>
</div>
}
}
#[component]
fn App() -> impl IntoView {
provide_meta_context();
view! {
<main>
<h1>"Rust is Awesome"</h1>
<Button />
</main>
}
}
fn main() {
mount_to_body(App)
}
You’ve now built a functional, stylish web application with Rust. This is just the beginning of your journey with Rust. Keep exploring and innovating to realize the full potential of your web development skills with Rust.