Welcome back to our series on building web apps with Rust! After laying the foundation in our first article, it’s time to dive deeper into a critical topic for any dynamic application: advanced state management.
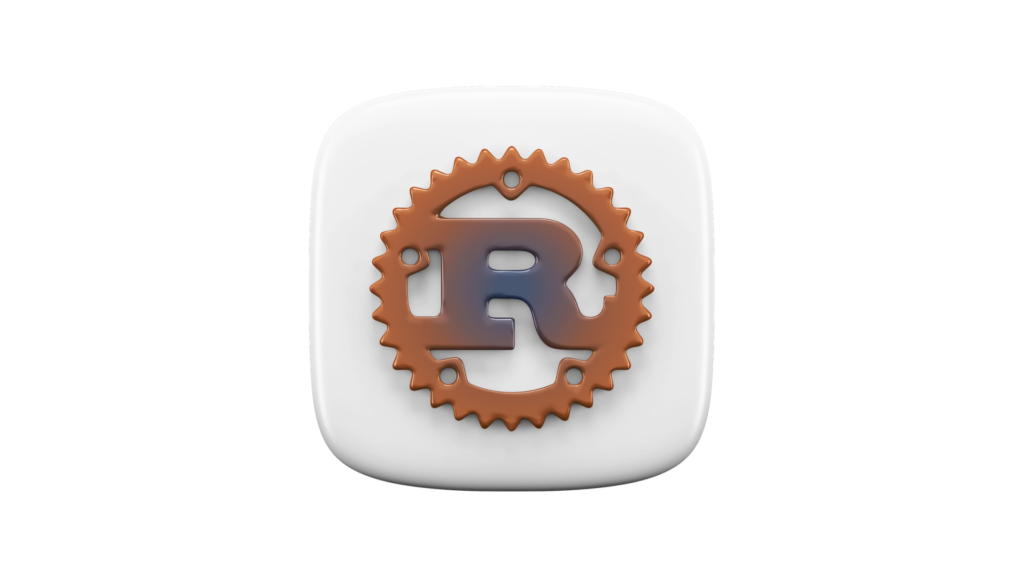
Why Focus on State Management?
State management is pivotal for keeping track of user interactions, data changes, and the overall behavior of your application. In Rust, managing state efficiently leverages the language’s strong type system and memory safety features to ensure data consistency and performance.
Exploring Libraries and Patterns
Several tools can help streamline state management in Rust web applications. Here’s a look at some of the most effective ones:
- Yew’s Context API: Ideal for passing state and behaviors through component hierarchies without prop drilling.
- Redux-style State Management: Inspired by Redux in the JavaScript ecosystem, this pattern uses a single, immutable state tree, making state predictable and easy to manage.
Practical Implementation: Building a Todo List in Rust
To put theory into practice, let’s develop a simple to-do list application implementing a Redux-style state management approach using the Yew framework, a popular choice for frontend development in Rust.
use yew::prelude::*;
use yewdux::prelude::*;
#[derive(Clone, Default, PartialEq, Store)]
struct State {
todos: Vec<String>,
}
struct App {
dispatch: Dispatch<BasicStore<State>>,
}
enum Msg {
AddTodo(String),
RemoveTodo(usize),
}
impl Component for App {
type Message = Msg;
type Properties = ();
fn create(ctx: &Context<Self>) -> Self {
Self {
dispatch: Dispatch::<BasicStore<State>>::new(),
}
}
fn update(&mut self, ctx: &Context<Self>, msg: Self::Message) -> bool {
match msg {
Msg::AddTodo(todo) => {
self.dispatch.reduce_mut(|state| state.todos.push(todo));
true
},
Msg::RemoveTodo(index) => {
self.dispatch.reduce_mut(|state| state.todos.remove(index));
true
},
}
}
fn view(&self, ctx: &Context<Self>) -> Html {
let todos = self.dispatch.get().todos.clone();
html! {
<>
<h1>{"Todo List"}</h1>
<input type="text" id="new-todo" onkeypress={ctx.link().batch_callback(|e: KeyboardEvent| {
if e.key() == "Enter" {
let input: HtmlInputElement = e.target_unchecked_into();
input.set_value("");
Some(Msg::AddTodo(input.value()))
} else {
None
}
})} />
<ul>
{ for todos.iter().enumerate().map(|(index, todo)| {
html! {
<li>
{ todo }
<button onclick={ctx.link().callback(move |_| Msg::RemoveTodo(index))}>{"Remove"}</button>
</li>
}
})}
</ul>
</>
}
}
}
fn main() {
yew::start_app::<App>();
}
This example demonstrates how to use Rust’s powerful features to manage state in a web environment effectively. The App
component handles user interface and dispatches actions to modify the state of the todo list.
The Rust’s Power in Web App Development
Advanced state management is a game-changer for Rust web app development, offering a pathway to build robust, efficient applications. By understanding and applying these concepts, you’re well on your way to mastering Rust web development.
Stay tuned for our next post, where we’ll explore integrating Rust web apps with backend services!