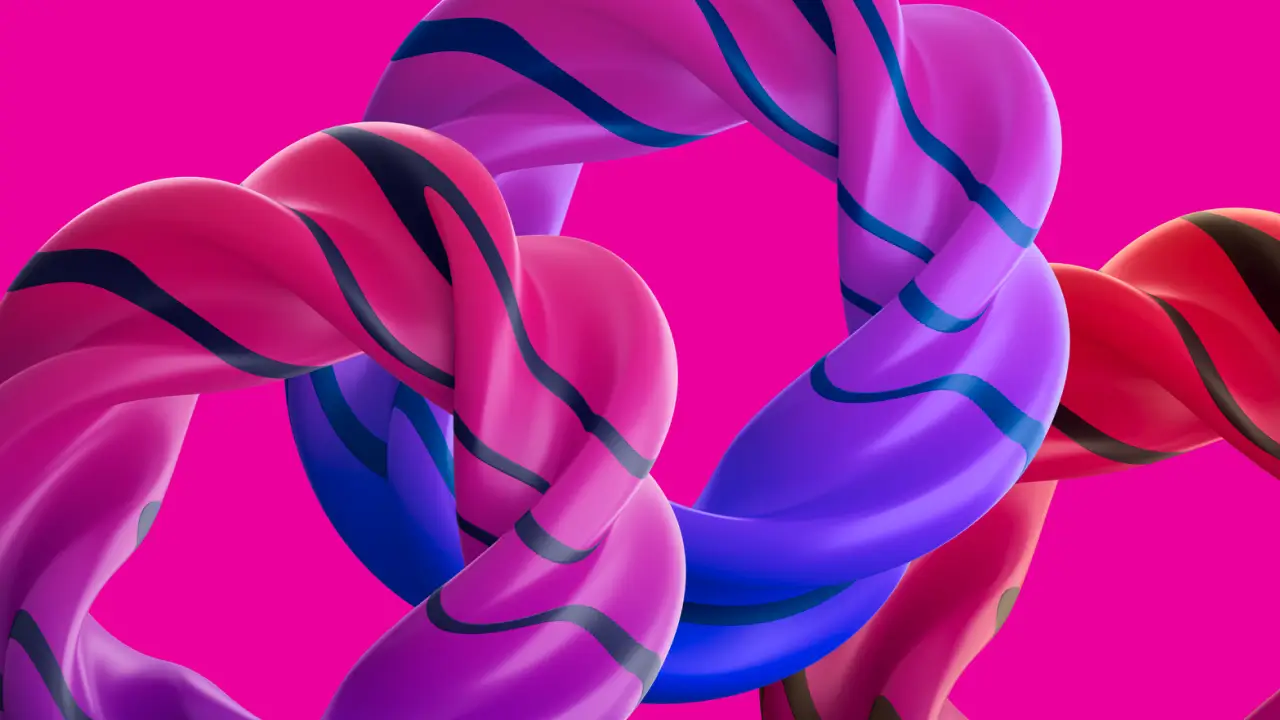
UX/UI
Catch Bugs Early with Prototyping: As a New Neo, Discover Your App’s WorldThe role of hi-fi prototyping in app development
April 29, 2024Chapter 3: Enhancing your Rust web app with powerful backend solutions
April 29, 2024
By: Michael Cardoza
Welcome back to our series on building web apps with Rust! In this installment, we’re diving into how to enhance your applications by integrating robust backend services. This guide will walk you through setting up and connecting Rust-based backend services, focusing on RESTful APIs and GraphQL.
When it comes to backend performance, Rust is unmatched, thanks to its emphasis on safety and speed. Tools like Rocket and Actix-Web allow developers to build reliable web servers that are not only resilient but also incredibly fast. This section will elaborate on how using Actix-Web can elevate your app’s performance through a RESTful API.
Actix-Web is a powerhouse among web frameworks due to its use of Rust’s asynchronous features and its safety guarantees. It’s designed to create highly scalable applications that can manage multiple requests at high speeds without compromising security. Here’s a more detailed setup for a basic RESTful services:
use actix_web::{web, App, HttpResponse, HttpServer, Responder};
async fn greet() -> impl Responder {
HttpResponse::Ok().body("Hello from your Rust Backend!")
}
pub fn main() {
let app = HttpServer::new(|| {
App::new().route("/", web::get().to(greet))
});
println!("Server running at http://localhost:8080/");
app.bind("0.0.0.0:8080").expect("Failed to bind server").run().await.unwrap();
}
In this snippet, the greet
function represents a simple endpoint that returns a welcoming message. This example demonstrates how straightforward it is to set up a basic web server with Actix-Web, allowing developers to focus more on application logic rather than boilerplate code.
GraphQL offers a significant advantage over RESTful APIs by allowing clients to request exactly the data they need. Rust’s powerful async capabilities make it an excellent match for GraphQL, enabling efficient and flexible data queries that can scale with user demands.
Async-graphql is a library tailored for creating GraphQL servers with Rust. It integrates seamlessly with frameworks like Actix-Web, providing a robust platform for developing GraphQL APIs. Below is an expanded example of setting up a basic GraphQL server:
use async_graphql::{Schema, Context, Object};
use async_graphql_actix_web::{GraphQLResponse, GraphQLRequest};
struct QueryRoot;
#[Object]
impl QueryRoot {
async fn hello(&self, ctx: &Context<'_>) -> String {
"Hello, GraphQL World!".to_string()
}
}
pub fn main() {
let schema = Schema::build(QueryRoot, EmptyMutation, EmptySubscription).finish();
println!("GraphQL server running at http://localhost:8000/graphql");
// Further code to integrate with Actix-web here
}
In this setup, QueryRoot
defines a simple GraphQL query that returns a greeting. This illustrates how Rust can be used to implement a GraphQL API that is not only performant but also concise and type-safe.
By integrating powerful backend services using Rust, your web applications will not only be robust but also incredibly performant and secure. Whether you’re setting up RESTful APIs or GraphQL, Rust has the capabilities to support complex and efficient backend architectures.
Stay tuned for our next chapter, where we delve into authentication and authorization techniques for your Rust web apps!