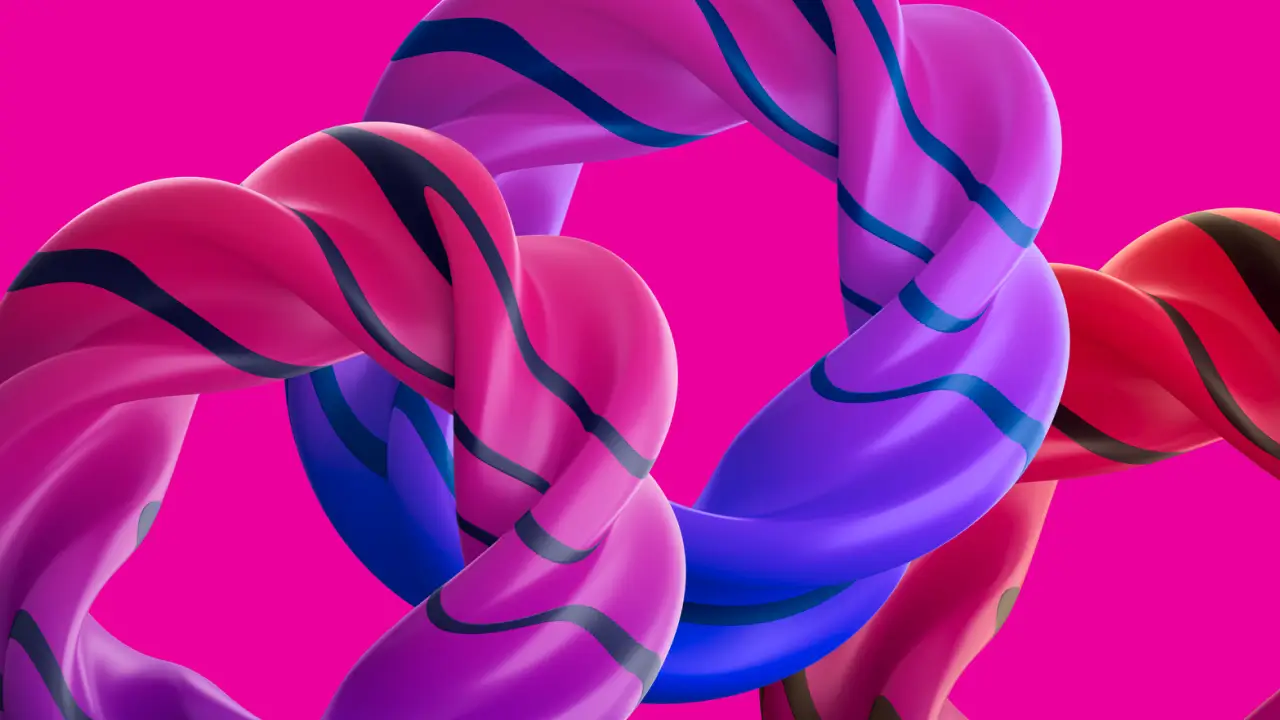
UX/UI
Aesthetics vs. Functionality: Can Beauty and Brains Coexist in Web Development?How to create compelling, user-friendly websites that stand out
May 6, 2024Chapter 4: Enhancing security in your Rust web apps
May 6, 2024
By: Maria
Welcome back to our series on building web apps with Rust! This chapter analyzes security mechanisms for web applications: authentication and authorization. We’ll explore implementing these using popular strategies like JWT and OAuth, ensuring your app handles user data securely.
JSON Web Tokens (JWT) are a compact and secure way of handling authentication and transferring information between parties. Here’s how you can implement JWT in Rust:
use jsonwebtoken::{encode, Header};
use serde::{Deserialize, Serialize};
#[derive(Debug, Serialize, Deserialize)]
struct Claims {
sub: String,
company: String,
exp: usize,
}
fn main() {
let my_claims = Claims { sub: "1234567890".to_string(), company: "ACME".to_string(), exp: 10000000000 };
let token = encode(&Header::default(), &my_claims, "secret".as_ref()).unwrap();
println!("Token: {}", token);
}
We’ll walk through generating and validating JWTs, explaining each step to ensure you understand how to apply these techniques in your own applications.
OAuth is a widely-used authorization protocol that enables applications to authenticate via external services such as Google, Facebook, and Twitter. Integrating OAuth in Rust involves using libraries like oauth2
to handle OAuth flows securely. It’s crucial to implement additional security measures such as CSRF protection using middleware that validates state parameters returned in OAuth responses.
Managing user sessions securely is critical in web applications. Rust offers several libraries, such as actix-session
for Actix Web, that facilitate encrypted and cookie-based session management. Best practices include:
HttpOnly
and Secure
flags to prevent access via JavaScript and ensure transmission over HTTPS.By implementing these advanced authentication and authorization techniques, your Rust web applications will not only be more secure but also prepared to handle complex user interactions safely and effectively. With a solid security foundation in place, it’s now the perfect time to focus on enhancing the efficiency and speed of your app.
Stay tuned as we explore how to optimize the performance of your Rust web apps in the next chapter. We’ll dive into efficient async programming, minimizing WebAssembly bundle size, and leveraging Rust’s powerful concurrency features to ensure your applications are not just secure, but blazing fast and responsive.