Welcome back to our in-depth series on Rust web apps development! Today, we analyze performance optimization, a crucial aspect to ensure that your web apps run smoothly and efficiently.
Efficient Async Programming in Rust
Asynchronous programming is essential for improving the responsiveness of your web applications. Rust’s async ecosystem allows you to handle multiple tasks concurrently without blocking the main thread, thereby enhancing performance.
Using async
in Rust can enhance the responsiveness of your web apps. Here’s a simple example of how to implement an asynchronous function to fetch data from a server:
use reqwest;
use tokio;
#[tokio::main]
async fn main() {
let response = reqwest::get("https://api.example.com/data")
.await
.unwrap()
.text()
.await
.unwrap();
println!("Response: {}", response);
}
Minimizing WebAssembly Bundle Size
WebAssembly plays a vital role in Rust web apps, especially for client-side operations. Optimizing your WebAssembly bundle size through techniques like tree shaking, code splitting, and utilizing efficient serialization can significantly speed up load times and improve user experience.
Optimizing the WebAssembly bundle size is crucial for performance. Below is a snippet demonstrating how you might use wasm-opt
to optimize the output:
wasm-opt -Oz -o optimized.wasm original.wasm
This command line tool improves the size and speed of your WebAssembly binary.
Leveraging Rust’s Concurrency Features
Rust offers powerful concurrency features that allow you to execute multiple operations in parallel safely. Harnessing these capabilities can reduce the execution time of CPU-bound tasks, making your application faster and more responsive.
Here’s how you might use Rust’s concurrency features to perform parallel computations:
use std::thread;
fn main() {
let handles: Vec<_> = (0..10).map(|_| {
thread::spawn(|| {
// perform some CPU-bound work here
let sum: u32 = (1..1000).sum();
sum
})
}).collect();
for handle in handles {
println!("Thread finished with count={}", handle.join().unwrap());
}
}
This example creates ten threads to execute computations in parallel, showcasing how concurrency can be utilized to enhance performance.
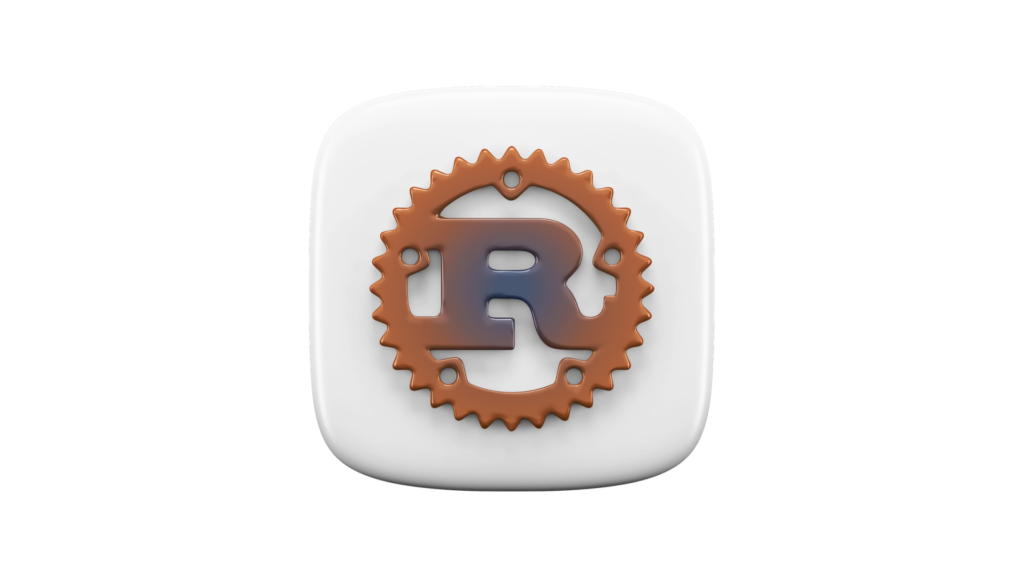
Conclusion
Optimizing the performance of your Rust web apps involves a combination of efficient coding practices, thoughtful application architecture, and leveraging the powerful features provided by Rust and WebAssembly. By implementing these strategies, you can ensure that your application is not only functional but also high-performing.
Stay tuned for our next chapter, where we’ll explore testing and debugging strategies for Rust web apps!