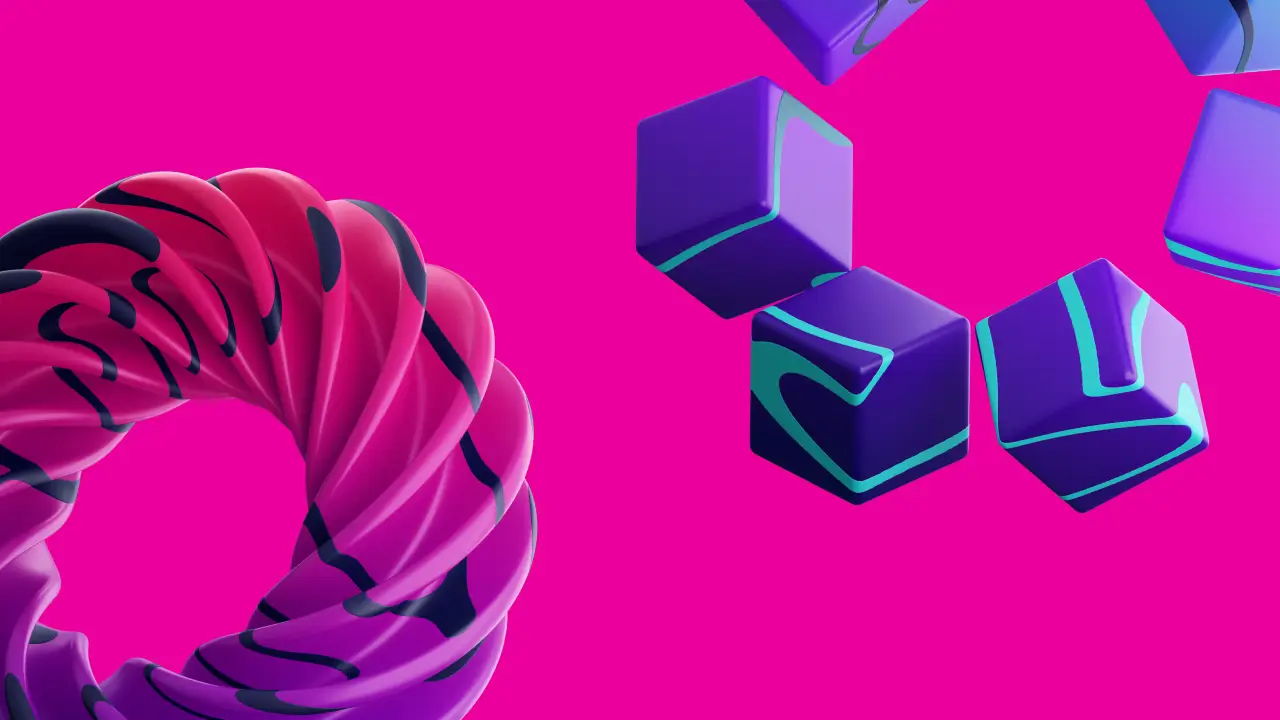
UX/UI
A Beginner’s Guide to Understanding User Interface (UI)Navigating the digital world one screen at a time
September 4, 2023Strumming the Code: Making Your JavaScript Sing
September 4, 2023
By: Michael Cardoza
Ever felt like your website’s as sluggish as a Monday morning without coffee? You’re not alone. The digital universe is loaded with websites struggling to hit the right notes of performance, often bogged down by clunky JavaScript files.
But worry not, fellow web maestros! If you enjoyed our Day.js JavaScript Date Management Guide, you’re in for another treat. We’re here to guide you through the process of JavaScript optimization, ensuring your website performs like a well-rehearsed orchestra.
We’ve all been there – those endless nights where coding feels like a hamster wheel. Loops, if not fine-tuned, can indeed feel like running in circles without a destination. Instead of the old-school for
loop, it’s high time you dance to the tunes of forEach
, map
, and filter
. They don’t just offer a clearer path but boost your code’s tempo.
Old Way: Traditional for
loop.
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = [];
for (let i = 0; i < numbers.length; i++) {
squaredNumbers.push(numbers[i] * numbers[i]);
}
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
New Rhythm: Using map
for clarity and speed.
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(number => number * number);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Ever thought of using the break
statement? It’s like hitting the pause button during a song, skipping needless repetitions. If you’re scratching your head, don’t just rely on hearsay. Check into developer forums and see these strategies in action.
Imagine a music band where every instrument wants to be the soloist. Chaos, right? Debouncing and throttling ensure your code’s components play in rhythm, not letting any function overshadow the others.
function debounce(func, delay) {
let timeout;
return (...args) => {
clearTimeout(timeout);
timeout = setTimeout(() => func(...args), delay);
};
}
const handleResize = () => {
// Perform resizing-related tasks
};
window.addEventListener('resize', debounce(handleResize, 300));
ES6 wasn’t just another update; it was like introducing a brand-new instrument to an orchestra. Data structures like Set
and Map
are those new beats that can redefine your coding rhythm. If your code was a sandwich, think of it as that special ingredient making it stand out!
For example, you can use Set
for unique values. It’s that special ingredient to make your code stand out!
const uniqueValues = new Set();
uniqueValues.add(1);
uniqueValues.add(2);
uniqueValues.add(1); // Won't be added again
console.log([...uniqueValues]); // [1, 2]
Changing from innerHTML
to textContent
isn’t about being whimsical; it’s about choosing the faster horse in the race. It’s like preferring acoustic to electric when you know the former fits the song better.
Using innerHTML
:
const element = document.getElementById('myElement');
element.innerHTML = '<strong>Updated content</strong>';
With textContent
:
const element = document.getElementById('myElement');
element.textContent = 'Updated content';
Who invited errors to this concert, anyway? But like that unexpected instrument going out of tune, errors happen. With try
, catch
, and finally
, you ensure the show goes on, and the audience (read: users) remains engrossed.
function parseJson(jsonString) {
try {
const parsedData = JSON.parse(jsonString);
return parsedData;
} catch (error) {
console.error('Error parsing JSON:', error.message);
return null;
}
}
const validJson = '{"name": "John", "age": 30}';
const invalidJson = 'invalid-json';
const validResult = parseJson(validJson);
console.log(validResult); // Output: { name: 'John', age: 30 }
const invalidResult = parseJson(invalidJson);
console.log(invalidResult); // Output: null
It’s like being a maestro, knowing where each note (event) fits. Rather than bombarding every section of the orchestra with cues, be selective. Choose a common anchor point and let the symphony flow organically.
Instead of attaching individual event listeners,
const buttons = document.querySelectorAll('.button');
buttons.forEach(button => {
button.addEventListener('click', handleClick);
});
Use event delegation on a parent element:
document.addEventListener('click', event => {
if (event.target.classList.contains('button')) {
handleClick(event);
}
});
Think of JavaScript optimization as composing a piece of music. Each tweak, every adjustment, harmonizes the output, making web interactions melodious for your audience. But remember, the world of web development is a vast ocean, and there’s always more to explore.