Have you ever wondered how apps send you updates even when you’re not using them? Whether it’s breaking news, a special discount, or a reminder, push notifications are a powerful tool for keeping users engaged. If you’re building a React Native app, you’ll definitely want to include them—but where do you start?
This chapter of React Native Unplugged will walk you through setting up push notifications using Firebase Cloud Messaging (FCM) for both Android and iOS. Let’s dive in and make sure your app stays connected with its users!
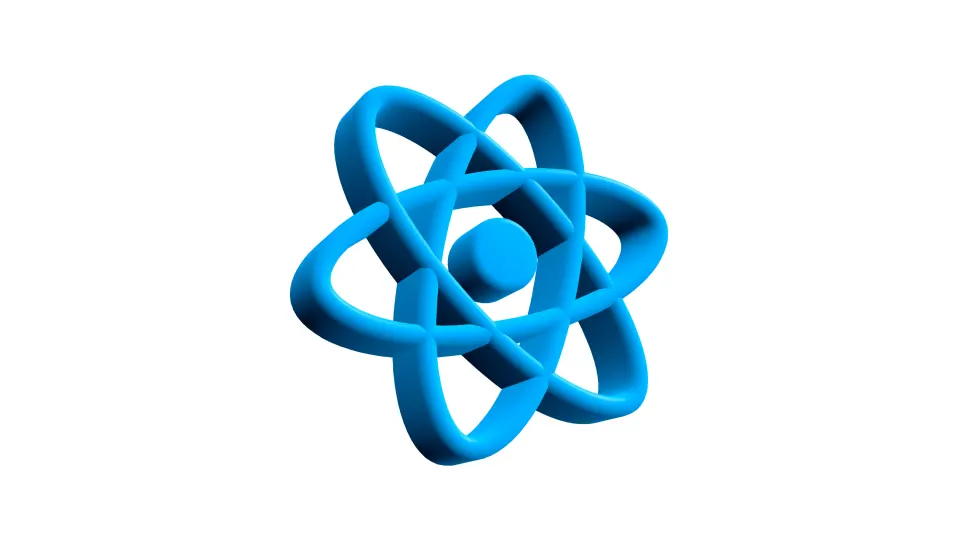
1. Set Up Firebase for Push Notifications
To enable push notifications, you need to integrate Firebase into your React Native project.
Step 1: Create a Firebase Project
- Go to Firebase Console.
- Click Add Project and follow the setup process.
- Once created, add an Android and/or iOS app to the project.
Step 2: Install Firebase Dependencies
Run the following command to install Firebase and related dependencies:
npm install @react-native-firebase/app @react-native-firebase/messaging
For iOS, install the pods:
cd ios pod install
2. Configure Firebase for Android
Step 1: Add Google Services JSON
- Download the
google-services.json
file from Firebase. - Place it inside
android/app/
.
Step 2: Modify android/build.gradle
Ensure that the following is included:
buildscript { dependencies { classpath("com.google.gms:google-services:4.3.10") } }
Step 3: Modify android/app/build.gradle
Add the following at the bottom:
apply plugin: 'com.google.gms.google-services'
3. Configure Firebase for iOS
Step 1: Add Google Services Info Plist
- Download
GoogleService-Info.plist
from Firebase. - Place it inside
ios/{your_project}/
.
Step 2: Modify ios/Podfile
Enable Firebase messaging by adding:
target 'YourProject' do use_frameworks! pod 'Firebase/Messaging' end
Then run:
cd ios pod install
Step 3: Enable Push Notifications in Xcode
- Open your project in Xcode.
- Go to Signing & Capabilities.
- Enable Push Notifications and Background Modes (select
Remote notifications
).
4. Request User Permissions
Before receiving push notifications, request user permissions.
import messaging from '@react-native-firebase/messaging'; async function requestUserPermission() { const authStatus = await messaging().requestPermission(); const enabled = authStatus === messaging.AuthorizationStatus.AUTHORIZED || authStatus === messaging.AuthorizationStatus.PROVISIONAL; if (enabled) { console.log('Notification permission granted.'); } } requestUserPermission();
5. Receive Push Notifications
Handle Foreground Notifications
import messaging from '@react-native-firebase/messaging'; import { Alert } from 'react-native'; messaging().onMessage(async remoteMessage => { Alert.alert('A new notification!', JSON.stringify(remoteMessage)); });
Handle Background and Quit State Notifications
import messaging from '@react-native-firebase/messaging'; messaging().setBackgroundMessageHandler(async remoteMessage => { console.log('Message handled in the background!', remoteMessage); });
6. Get Device Token for Sending Notifications
import messaging from '@react-native-firebase/messaging'; async function getDeviceToken() { const token = await messaging().getToken(); console.log('FCM Device Token:', token); } getDeviceToken();
This token is used to send push notifications to specific devices.
7. Send Push Notifications via Firebase Cloud Messaging
You can send push notifications using Firebase’s REST API.
Make a POST Request to Firebase Cloud Messaging
curl -X POST "https://fcm.googleapis.com/fcm/send" \ -H "Authorization: key=YOUR_SERVER_KEY" \ -H "Content-Type: application/json" \ -d '{ "to": "DEVICE_FCM_TOKEN", "notification": { "title": "Hello!", "body": "This is a test notification" } }'
Replace YOUR_SERVER_KEY
with your Firebase server key and DEVICE_FCM_TOKEN
with the token retrieved earlier.
8. Handling Notification Clicks (Deep Linking)
If you want to navigate users to a specific screen when they click a notification:
import { useEffect } from 'react'; import messaging from '@react-native-firebase/messaging'; import { useNavigation } from '@react-navigation/native'; const App = () => { const navigation = useNavigation(); useEffect(() => { messaging().onNotificationOpenedApp(remoteMessage => { navigation.navigate(remoteMessage.data.screen); }); messaging() .getInitialNotification() .then(remoteMessage => { if (remoteMessage) { navigation.navigate(remoteMessage.data.screen); } }); }, []); };
9. Testing Push Notifications Locally
Use Firebase’s testing tool:
- Go to Firebase Console → Cloud Messaging.
- Click Send Your First Message.
- Enter a test message and send it to your registered device token.
10. Conclusion
Push notifications are a must-have feature for any modern mobile app, keeping users engaged and informed. By integrating Firebase Cloud Messaging, you can easily send targeted notifications to both Android and iOS devices.
Push notifications? Check. Up next: slick animations in React Native that’ll bring your app to life.