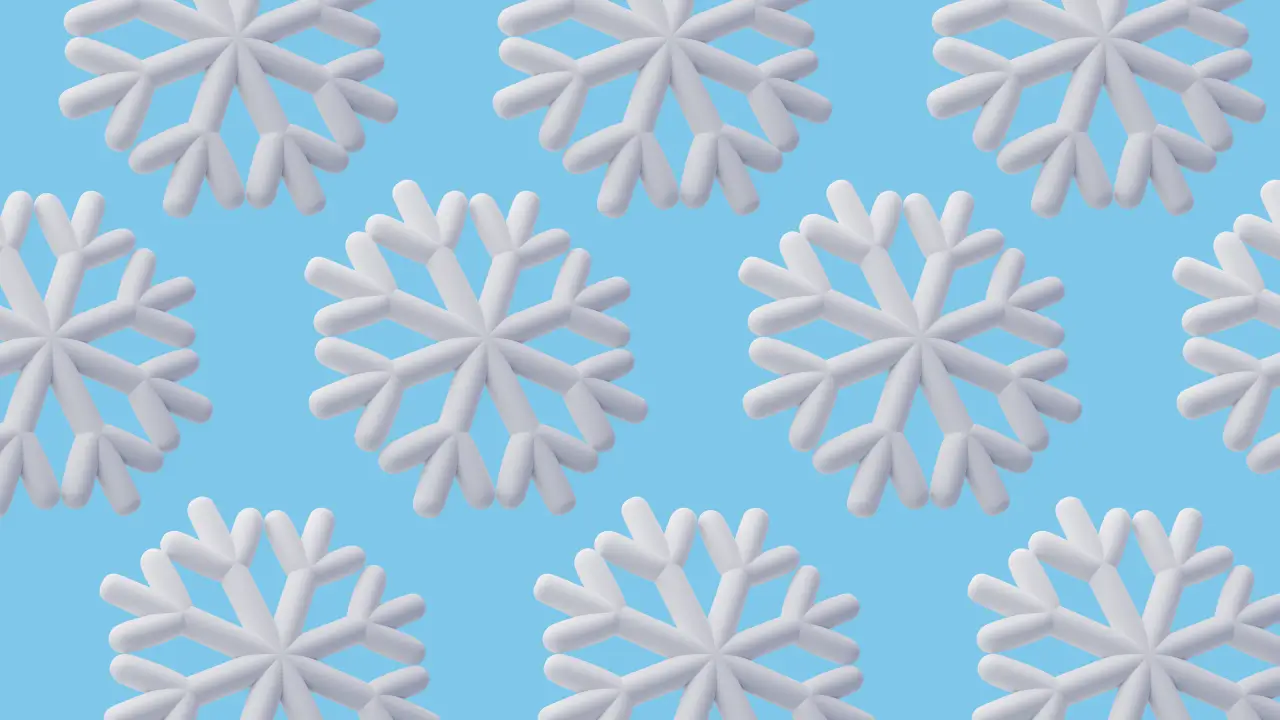
Development
From Confusion to Solution: How We Removed Hidden Malware from our WebsiteExploiting popular CDNs: A trap we didn’t see coming
June 24, 2024Simplify your coding and boost efficiency!
June 24, 2024
By: Enrique
Ever tried to get specific items out of an array and ended up feeling like a cat chasing a laser pointer? Fear not! Here’s a neat trick to make your life easier. You can actually destructure an array by its indices. It’s like having a magic wand that extracts only the values you want.
For instance, if you have an array and only need two values from it, you can do this:
const { 0: designer, 3: developer } = [
"Marcos",
"Rene",
"Diego",
"Michael",
"Enrique"
];
console.log({ designer, developer });
// Output: { designer: 'Marcos', developer: 'Michael' }
Easy-peasy, right? Just like ordering only the toppings you want on your pizza. No more endless loops or confusing logic—just straightforward code that gets the job done.
JavaScript tests can sometimes feel like untangling a pair of headphones (why are they always tangled?!). One common problem is checking if an array has duplicate elements. Many devs dive headfirst into loops and logic, but here’s a quick fix that’s as refreshing as a cold lemonade on a hot day.
Consider these arrays:
const a = [1, 2, 3, 2, 3, 2, 4, 5];
const b = ["Sofia", "Enrique", "Marcos", "Fabian"];
const c = [9, 5, 4, 1, 0];
You can check for duplicates with a simple function:
const hasDuplicateValues = (array) => {
return new Set(array).size < array.length;
}
console.log(hasDuplicateValues(a)); // true
console.log(hasDuplicateValues(b)); // false
console.log(hasDuplicateValues(c)); // false
Here’s the trick: You create a Set
from your array. Since Set
only keeps unique values, its size will be smaller if there were duplicates. Think of it as a bouncer at a club—no duplicate entries allowed!
Using these tricks not only makes your code cleaner but also more efficient. Destructuring arrays by indices can save you from writing tedious, repetitive code. Checking for duplicates with a Set
is not only elegant but also faster than using loops or other complex logic.
And there you have it! Two simple yet powerful tricks to make your JavaScript coding life a breeze. Whether you’re pulling specific items from an array or ensuring there are no duplicate entries, these techniques will save you time and headaches. Now go forth and code with confidence, knowing you’ve got these handy tricks up your sleeve!