How well do you really understand state management in React Native? Do you know when to use Redux and when the Context API is a better fit? Or are you just following whatever guide pops up first on Google? State management can be the backbone of a smooth and scalable app, but picking the right solution is crucial. Luckily, this chapter of React Native Unplugged is here to break it all down and help you choose the best approach for your project.
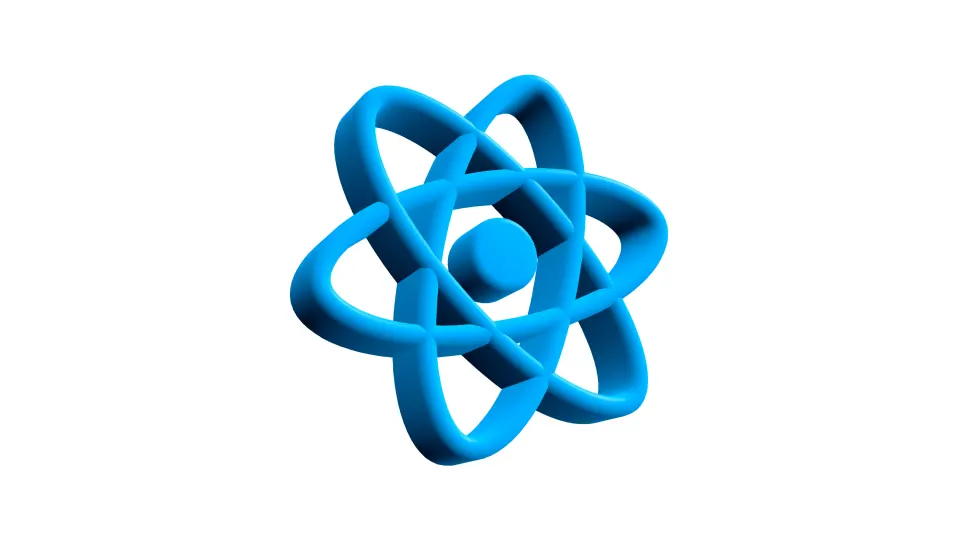
1. Introduction to State Management
React Native components have local state, but as your application grows, managing state across multiple components becomes challenging. This is where state management solutions like Redux and the Context API become useful.
2. Redux: A Predictable State Container
Redux is a widely used library for managing application state in a predictable way. It follows a unidirectional data flow and centralizes the state of the application in a single store.
Pros of Redux:
- Centralized state management
- Predictable state updates
- Large ecosystem with middleware like Redux Thunk and Redux Saga
- Excellent debugging support with Redux DevTools
Cons of Redux:
- Requires more boilerplate code
- Has a learning curve for beginners
- Can be excessive for small projects
Installing Redux in React Native
To install Redux and React-Redux, run:
npm install @reduxjs/toolkit react-redux --save
Setting Up Redux in a React Native App
- Create a Redux Store (
store.js
):
import { configureStore } from '@reduxjs/toolkit'; import counterReducer from './counterSlice'; export const store = configureStore({ reducer: { counter: counterReducer, }, });
- Create a Redux Slice (
counterSlice.js
):
import { createSlice } from '@reduxjs/toolkit'; const counterSlice = createSlice({ name: 'counter', initialState: { value: 0 }, reducers: { increment: (state) => { state.value += 1; }, decrement: (state) => { state.value -= 1; }, }, }); export const { increment, decrement } = counterSlice.actions; export default counterSlice.reducer;
- Provide the Store to the App (
App.js
):
import React from 'react'; import { Provider } from 'react-redux'; import { store } from './store'; import CounterScreen from './CounterScreen'; export default function App() { return ( <Provider store={store}> <CounterScreen /> </Provider> ); }
- Using Redux in a Component (
CounterScreen.js
):
import React from 'react'; import { View, Text, Button } from 'react-native'; import { useSelector, useDispatch } from 'react-redux'; import { increment, decrement } from './counterSlice'; export default function CounterScreen() { const count = useSelector((state) => state.counter.value); const dispatch = useDispatch(); return ( <View> <Text>Count: {count}</Text> <Button title="Increment" onPress={() => dispatch(increment())} /> <Button title="Decrement" onPress={() => dispatch(decrement())} /> </View> ); }
Performance Optimization in Redux
To avoid unnecessary re-renders in Redux, use useSelector
efficiently:
import { shallowEqual, useSelector } from 'react-redux'; const count = useSelector((state) => state.counter.value, shallowEqual);
This ensures the component only re-renders when the state actually changes.
3. Context API: A Simpler Alternative
It is best suited for simpler state management needs.
Performance Optimization in Context API
To prevent unnecessary re-renders, wrap the context value inside useMemo
:
const value = useMemo(() => ({ count, setCount }), [count]);
This ensures that the value only updates when count
changes, improving performance.
React’s Context API provides a built-in way to manage state without requiring third-party libraries. It is best suited for simpler state management needs.
Pros of Context API:
- No external dependencies
- Simpler setup compared to Redux
- Ideal for small to medium-sized applications
Cons of Context API:
- Performance issues in large applications
- No built-in middleware like Redux
- More complex state updates require additional logic
Using Context API in React Native
Create a Context Provider (CounterContext.js
):
import React, { createContext, useState, useMemo } from 'react'; export const CounterContext = createContext(); export const CounterProvider = ({ children }) => { const [count, setCount] = useState(0); const value = useMemo(() => ({ count, setCount }), [count]); return ( <CounterContext.Provider value={value}> {children} </CounterContext.Provider> ); };
4. Redux vs. Context API: Which One to Choose?
Feature | Redux | Context API |
---|---|---|
Complexity | High (requires setup) | Low (easy to use) |
Performance | Optimized with middleware | May cause unnecessary re-renders |
Scalability | Suitable for large apps | Best for small to medium apps |
Middleware Support | Yes (Thunk, Saga) | No |
Setup Time | Longer | Shorter |
Can You Use Both Redux and Context API?
Yes! Many large applications use Redux for global state (e.g., authentication, user preferences) and Context API for local component state (e.g., theming, modal visibility). This hybrid approach can improve performance while keeping your app scalable.
5. Conclusion
- Use Redux if your app is large, requires middleware, and needs predictable state management.
- Use Context API for smaller apps where state management is simple.
- Consider using both if your app benefits from local and global state separation.
Which state management solution do you prefer? Let us know in the comments! 🚀
Stay tuned for the next chapter in React Native Unplugged, where we’ll dive into another key topic to help you build better apps. You won’t want to miss it!