Doubt:
Why does this
behave differently depending on how a function is called?
Insight: Understanding this
in Different Contexts
this
In JavaScript,
refers to the execution context, which changes based on how and where a function is called. This dynamic behavior can be confusing, especially for developers transitioning from languages where this
this
behaves more predictably.
Here’s how this
behaves in different contexts:
In Regular Functions
this
is determined by the call context, meaning the object (or lack of object) that calls the function.- If the function is called globally or without an object,
this
defaults to the global object (window
in browsers orglobal
in Node.js). Instrict mode
,this
isundefined
.
In Methods
- When a function is called as a method of an object,
this
refers to the object the method belongs to.
In Arrow Functions
- Arrow functions do not have their own
this
. Instead, they inheritthis
from their enclosing lexical scope, making them useful for nested functions.
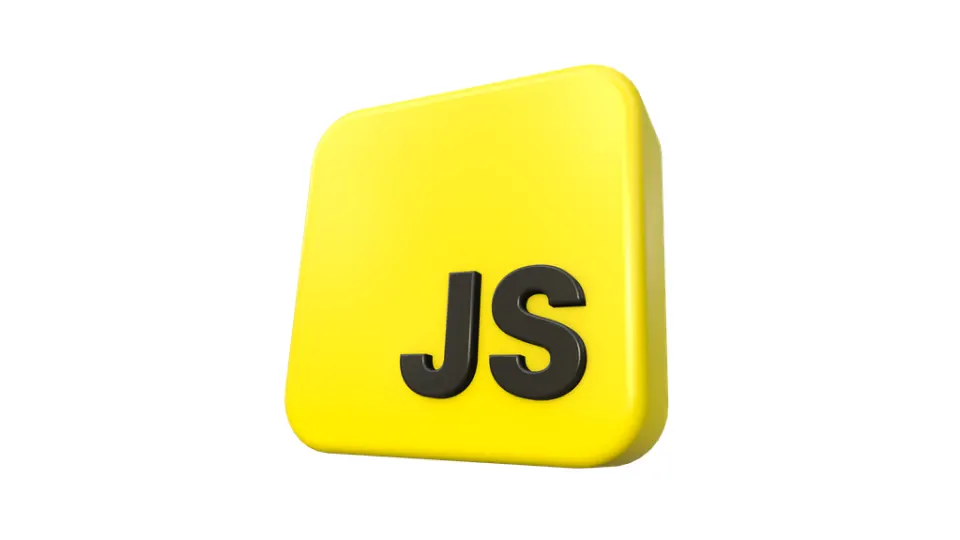
Example Code
Let’s use Tony Stark and his AI assistant, Jarvis, to illustrate how this
behaves in various contexts.
Global Context (Regular Function)
In a global function, this
depends on whether strict mode
is enabled.
function whoAmI() { console.log(this); // In non-strict mode, this is the global object (window/global) } whoAmI(); // Outputs: global object or undefined in strict mode
Method Context
When this
is used in a method, it refers to the object the method belongs to.
const jarvis = { name: "Jarvis", getName: function () { console.log(this.name); // 'this' refers to the jarvis object } }; jarvis.getName(); // Outputs: "Jarvis"
Nested Function (Problematic Context)
In regular nested functions, this
defaults to the global context because the nested function isn’t called as a method of the object.
const suit = { model: "Mark XLVII", getSuitDetails: function () { function showModel() { console.log(this.model); // 'this' refers to the global object (window/global), not the suit object } showModel(); } }; suit.getSuitDetails(); // Outputs: undefined (in strict mode) // or a global object's 'model' (if defined) in non-strict mode
Arrow Function (Solution for Nested Functions)
Arrow functions inherit this
from their surrounding scope, solving the issue in nested functions.
const suitFixed = { model: "Mark XLVII", getSuitDetails: function () { const showModel = () => { console.log(this.model); // 'this' is inherited from getSuitDetails's context }; showModel(); } }; suitFixed.getSuitDetails(); // Outputs: "Mark XLVII"
Why This Matters
- Global Confusion: In a global context or with nested functions,
this
may not point to the object you expect, leading to hard-to-debug issues. - Predictable Contexts: Using arrow functions ensures that
this
remains consistent in nested functions, while regular functions allowthis
to point to the object calling the method.
Best Practices for Managing this
- Use Arrow Functions for Lexical
this
:
Arrow functions are ideal for ensuring consistent context in nested functions since they inheritthis
from the enclosing scope. - Choose Regular Functions for Object Methods:
Regular functions are suited for object methods wherethis
should refer to the object calling the method. - Explicitly Set Context When Needed:
Usebind
,call
, orapply
to manually controlthis
when functions are called in non-method contexts.bind
: Creates a new function withthis
explicitly set.call
: Calls a function withthis
and arguments specified explicitly.apply
: Similar tocall
but accepts arguments as an array.
const suit = { model: "Mark XLVII", showModel: function () { console.log(this.model); } }; const showModelBound = suit.showModel.bind(suit); // Explicitly bind 'this' to suit showModelBound(); // Outputs: "Mark XLVII"
Final Takeaway
Understanding this
is critical for writing predictable and maintainable code. Think of this
as the object in focus—use arrow functions when inheriting the surrounding context makes sense, and regular functions for object methods where this
should refer to the object itself.
Ready to explore the future of JavaScript? Check out our breakdown of Promises and async/await to master asynchronous programming.