If you’ve ever felt like React Native is playing a cruel joke on you with cryptic error messages, you’re not alone. The dreaded react-native run-android
error can strike when you least expect it, turning your smooth development process into a debugging marathon. But fear not! This guide will walk you through the most common issues and how to fix them so you can get back to building awesome apps.
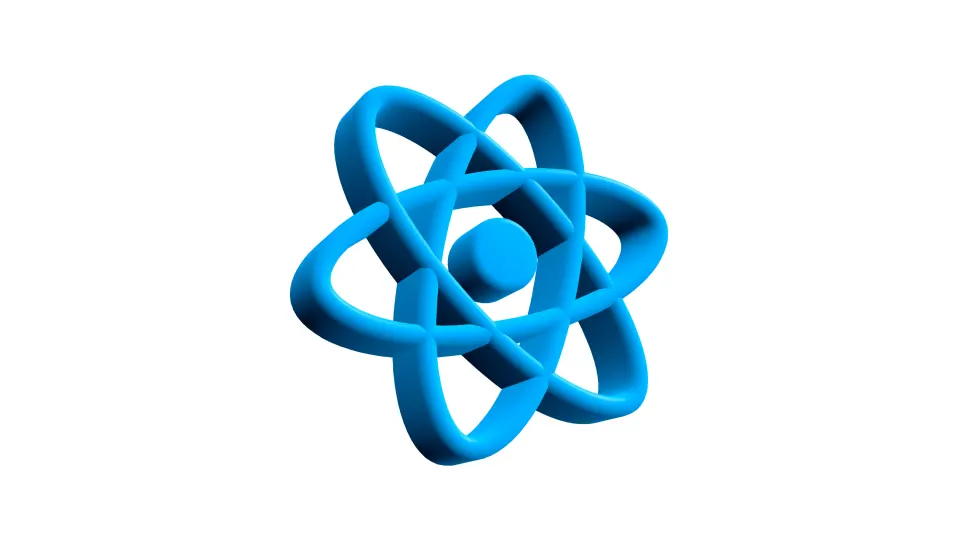
1. Verify Your Android Development Environment
Before running react-native run-android
, make sure your system is properly set up for Android development.
Ensure the following are installed:
- Node.js (Latest LTS version recommended)
- Use
npx react-native init ProjectName
to create projects. - Java Development Kit (JDK) (Java 11 recommended for newer React Native versions)
- Android SDK & Android Studio
- Android Virtual Device (AVD) or a connected device
- Proper PATH configuration
To check your setup, run:
npx react-native doctor
This command will flag any setup issues and suggest solutions.
2. Ensure an Android Device or Emulator is Running
Run the following command:
adb devices
If no devices appear, either start an emulator or connect an Android device with USB debugging enabled.
To manually start an emulator:
- Open Android Studio
- Navigate to AVD Manager (Tools > AVD Manager)
- Launch an emulator
Alternatively, run:
npx react-native start
npx react-native run-android
This helps isolate Metro Bundler issues from Gradle-related errors.
3. Fix Gradle Issues
Gradle build failures are common. Try the following fixes:
Clear Gradle Cache:
cd android && ./gradlew clean
Then rerun:
cd .. && npx react-native run-android
Upgrade Gradle Version Carefully
Instead of arbitrarily updating Gradle, check the recommended Gradle version for your React Native version:
cd android && ./gradlew --version
Then update android/build.gradle
only if necessary:
classpath("com.android.tools.build:gradle:7.0.2") // Example version
To update the Gradle wrapper:
cd android && ./gradlew wrapper --gradle-version 7.0.2 --distribution-type all
4. Resolve Dependency Conflicts
If you encounter dependency-related errors, run:
cd android && ./gradlew dependencies
Look for conflicting versions.
If necessary, update dependencies in android/app/build.gradle
and package.json
, then reinstall node modules:
rm -rf node_modules
rm -rf android/app/build
npm install
npx react-native run-android
5. Fix Java/Kotlin Compatibility Issues
Ensure Java and Kotlin versions match your Gradle settings. Check android/gradle.properties
:
org.gradle.java.home=/path/to/your/java/sdk
If using Kotlin, update build.gradle
:
ext.kotlin_version = '1.6.10'
6. Restart Metro Bundler
If the Metro Bundler crashes, reset and restart it:
npx react-native start --reset-cache
Then rerun your project:
npx react-native run-android
7. Fix Port & Network Conflicts
If another process is using port 8081, try this:
npx react-native start --port=8082
Or manually terminate processes using port 8081:
lsof -i :8081 # macOS/Linux
kill -9
For Windows:
taskkill /F /IM node.exe
8. Ensure ANDROID_HOME
is Set Correctly
If adb devices
returns no output, set the ANDROID_HOME
environment variable:
export ANDROID_HOME=$HOME/Library/Android/sdk # macOS
export ANDROID_HOME=$HOME/Android/Sdk # Linux
On Windows, set it via System Properties > Environment Variables.
9. Reinstall React Native & Rebuild the Project
If nothing works, reinstall React Native and rebuild:
rm -rf node_modules
npm install
cd android && ./gradlew clean
cd .. && npx react-native run-android
Conclusion
Fixing react-native run-android
errors may feel like untangling a ball of wires in the dark, but with the right approach, you’ll be back on track in no time. By systematically addressing dependencies, Gradle configurations, and environment issues, you can conquer the chaos and keep your development flow smooth.
If this guide helped, stay tuned for more troubleshooting tips in React Native Unplugged—because let’s be real, React Native always has a few more surprises up its sleeve!