You can write Python, but can you write beautiful Python? Just like any language, code can be messy and chaotic, or it can be clean, structured, and easy to follow. Writing beautiful Python is about writing code that’s as fluid and readable as any well-spoken language. Here are five tips to help you make your Python code not just functional, but elegant and Pythonic.
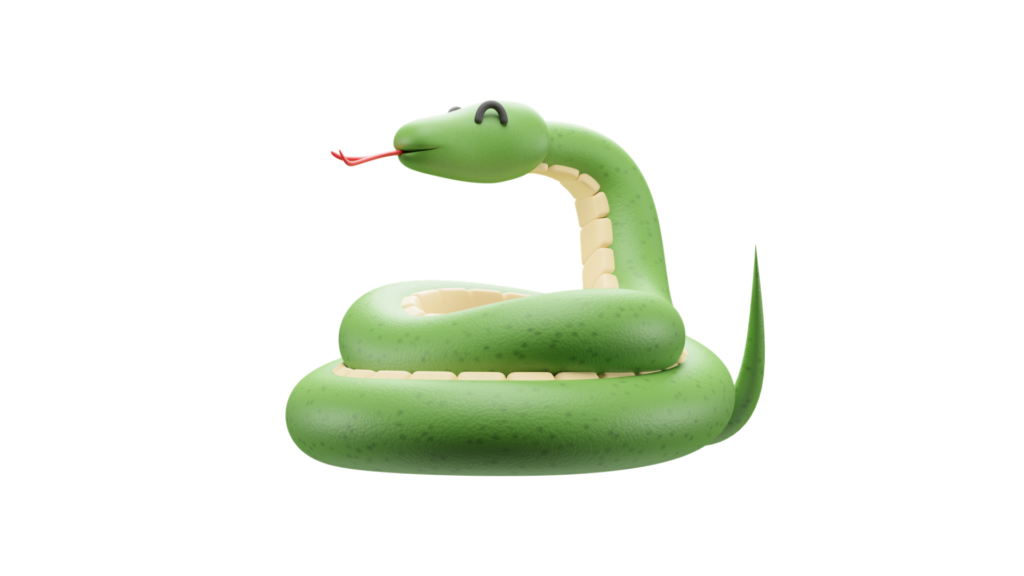
1. Embrace PEP 8, the Style Guide for Python Code
Think of PEP 8 as the grammar book for Python. It provides guidelines for formatting your code, making it consistent and easy to read. Here are some key points:
- Use 4 spaces for indentation (no tabs)
- Limit lines to 79 characters
- Use blank lines to separate functions and classes
- Follow naming conventions:
snake_case
for functions and variables,CamelCase
for classes
# Not so beautiful: Non-PEP 8 compliant, unclear naming
def CALCULATE_sum(x,y):
return x+y
# Beautiful Python: PEP 8 compliant with proper naming and spacing
def calculate_sum(x, y):
return x + y # Notice the spaces around the operator
2. Choose Descriptive Names
In beautiful Python, names should be descriptive and meaningful. This makes your code self-explanatory and reduces the need for comments.
# Unclear: Non-descriptive names make it hard to understand what the function does
def f(a, b):
return a - b
# Clear and descriptive: Descriptive names (minuend, subtrahend) make the function's purpose obvious
def subtract(minuend, subtrahend):
return minuend - subtrahend
3. Keep It Simple and Straightforward
Beautiful Python embraces simplicity. Avoid unnecessary complexity and stick to the “Explicit is better than implicit” principle.
# Overly complex: The conditional expression is unnecessary
def is_even(num):
return True if num % 2 == 0 else False
# Simple and beautiful: The conditional can be simplified
def is_even(num):
return num % 2 == 0 # Directly return the result of the condition
4. Leverage List Comprehensions and Generator Expressions
Python offers elegant ways to work with sequences. List comprehensions and generator expressions can make your code more concise and readable.
# Traditional approach: Using a loop to build a list
squares = []
for i in range(10):
squares.append(i ** 2)
# Beautiful Python using list comprehension: More concise and Pythonic
squares = [i ** 2 for i in range(10)] # Generates the same list in a single, readable line
# Memory-efficient generator expression: Similar to list comprehension but doesn't store the entire list in memory
squares_gen = (i ** 2 for i in range(10)) # Useful for large datasets
5. Utilize Python’s Built-in Functions and Libraries
Python comes with “batteries included” β a rich set of built-in functions and libraries. Using these can make your code more efficient and Pythonic.
# Reinventing the wheel: Manual sum calculation using a loop
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num
# Beautiful Python using built-in function: sum() simplifies the task
total = sum(numbers) # Built-in function that efficiently sums the list
# Using enumerate for elegant iteration: More readable and efficient when you need both index and value
for index, value in enumerate(numbers):
print(f"Index {index}: {value}")
# enumerate() returns both the index and the value, eliminating the need for manual index tracking
Conclusion: Write Python, But Write It Beautifully
Writing beautiful Python is an art that combines clarity, simplicity, and efficiency. By following these tips, you’ll create code that’s not just functional, but a joy to read and maintain.
So, the next time you sit down to code, ask yourself: “Am I just writing Python, or am I writing beautiful Python?” With these guidelines in mind, you’re well on your way to creating Python code that’s as elegant as it is effective.