Have you ever wondered why your React Native app feels sluggish? Are your animations lagging, load times dragging, or screens freezing up? It’s frustrating, especially when you’ve worked hard to build a smooth user experience. But don’t worry—this chapter of React Native Unplugged is here to help you identify the culprits behind slow performance and show you how to fix them. Let’s dive in and make your app run like a well-oiled machine!
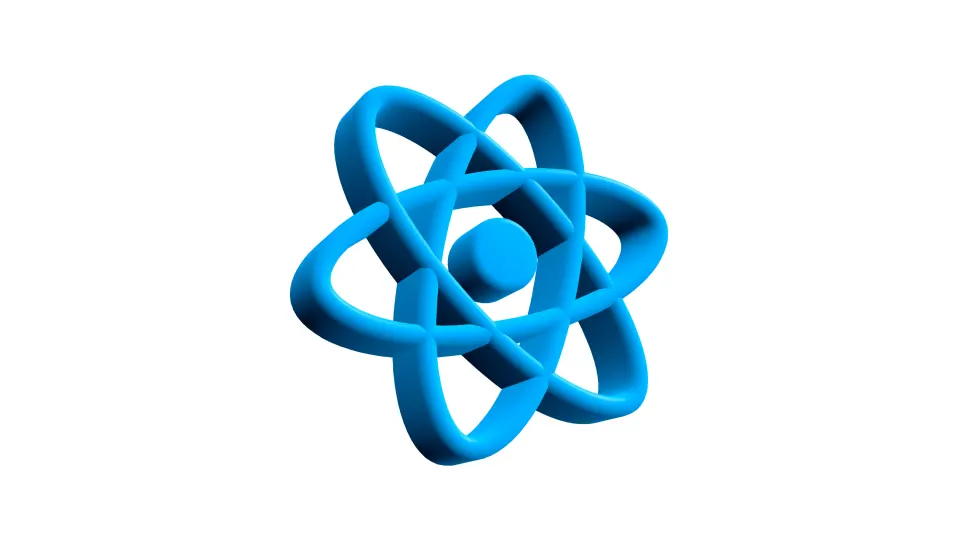
1. Common Causes of Slow Performance
Several factors can contribute to a slow React Native app:
- Unoptimized rendering: Excessive re-renders of components.
- Heavy JavaScript execution: Blocking the main thread with long-running tasks.
- Memory leaks: Improper cleanup of resources.
- Large image sizes: Uncompressed or oversized images.
- Inefficient state management: Poorly structured app state.
- Bridging overhead: Frequent communication between JavaScript and native code.
2. Performance Optimization Tips
1. Optimize Rendering with useMemo
, useCallback
, and React.memo
React Native re-renders components when state or props change. Unnecessary re-renders can slow down your app.
- Use
React.memo
to prevent unnecessary re-renders:
import React, { memo } from 'react'; const MyComponent = memo(({ value }) => { console.log("Rendering MyComponent"); return <Text>{value}</Text>; });
- Use
useMemo
to memoize complex calculations:
const expensiveCalculation = useMemo(() => { return heavyFunction(input); }, [input]);
- Use
useCallback
to memoize functions passed as props:
const handleClick = useCallback(() => { console.log("Button clicked"); }, []);
2. Reduce JavaScript Thread Work
Avoid long-running JavaScript tasks that block the main thread.
- Solution: Use
setTimeout
,requestAnimationFrame
, or move heavy tasks to a background thread using libraries like react-native-worker or react-native-reanimated.
Example with react-native-reanimated
:
import Animated, { useSharedValue, withSpring } from 'react-native-reanimated'; const offset = useSharedValue(0); const moveBox = () => { offset.value = withSpring(100); };
3. Optimize Images
Large images can significantly slow down the app, especially on lower-end devices.
- Solutions:
- Use compressed images (JPEG/WEBP instead of PNG).
- Use react-native-fast-image for efficient image loading.
- Avoid full-resolution images for thumbnails.
Example using react-native-fast-image
:
import FastImage from 'react-native-fast-image'; <FastImage style={{ width: 100, height: 100 }} source={{ uri: 'https://example.com/image.jpg' }} />
4. Prevent Memory Leaks
Memory leaks can slow down the app and lead to crashes.
- Solutions:
- Unsubscribe from listeners in
useEffect
:
- Unsubscribe from listeners in
useEffect(() => { const subscription = someEvent.subscribe(() => {}); return () => subscription.unsubscribe(); }, []);
- Use WeakMap for caching.
- Avoid holding large objects in state.
5. Efficient State Management
Poorly managed state can lead to unnecessary re-renders and performance bottlenecks.
- Solutions:
- Use Context API or Redux Toolkit for global state.
- Keep component-level state lightweight.
- Use Recoil or Zustand for performance-friendly state management.
Example with Zustand:
import create from 'zustand'; const useStore = create((set) => ({ count: 0, increment: () => set((state) => ({ count: state.count + 1 })), })); const Counter = () => { const { count, increment } = useStore(); return ( <View> <Text>{count}</Text> <Button title="Increment" onPress={increment} /> </View> ); };
6. Optimize the JavaScript-Native Bridge
React Native communicates between JavaScript and native code using a bridge. Excessive communication can slow down the app.
- Solutions:
- Batch bridge calls.
- Avoid passing large data objects through the bridge.
- Use react-native-reanimated for animations, as it runs on the UI thread without bridge communication.
7. Optimize Navigation Performance
Navigation transitions can feel sluggish if not optimized.
- Solutions:
- Use React Native Screens with
react-navigation
for faster screen rendering. - Enable
enableScreens
:
- Use React Native Screens with
import { enableScreens } from 'react-native-screens'; enableScreens();
8. Reduce App Size and Startup Time
Large app bundles increase load time.
- Solutions:
- Use Hermes Engine for faster JavaScript execution.
- Enable Proguard in Android to reduce APK size.
- Split APK by architecture (
arm64-v8a
,x86
).
Enable Hermes in android/app/build.gradle
:
project.ext.react = [ enableHermes: true, ]
9. Monitor Performance
Use performance monitoring tools to identify bottlenecks:
- React Native Performance Monitor: Press
Cmd+M
(Android) orCmd+D
(iOS) and enable the performance monitor. - Flipper: React Native debugging tool with performance plugins.
- Sentry/Crashlytics: For crash and performance tracking.
3. Conclusion
Performance issues can turn an otherwise great app into a frustrating experience for users. But the good news? You don’t have to settle for slow load times, laggy animations, or memory leaks. By optimizing rendering, managing state efficiently, reducing bridge communication, and keeping an eye on performance metrics, you can deliver a smooth, responsive app.
So, which of these tips will you try first? Start with the ones that align with your app’s pain points and build from there. And don’t forget—this is just one piece of the puzzle. Stay tuned for the next chapter of React Native Unplugged, where we’ll tackle another challenge and help you level up your development game. See you there!