How well do you really understand navigation in React Native? Do you know the difference between JavaScript-based and native navigation? Or are you just rolling with whatever tutorial you found first? Navigation is one of those things that can make or break your app’s user experience, and choosing the right approach is crucial. But don’t worry—this chapter of React Native Unplugged is here to answer all your questions and help you navigate (pun intended) the best solution for your project.
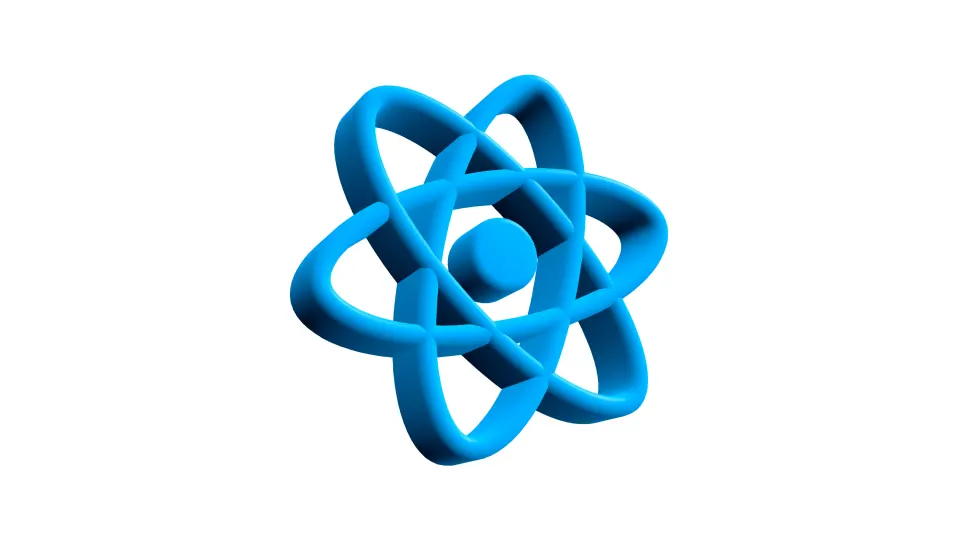
1. Understanding Navigation in React Native
Navigation enables users to move between different screens in an app. Since React Native doesn’t come with built-in navigation, developers rely on third-party libraries like React Navigation and React Native Navigation (Wix Engineering) to handle this functionality.
2. React Navigation (JavaScript-Based Navigation)
React Navigation is the most widely used navigation library in the React Native ecosystem. It is implemented in JavaScript and offers flexibility while ensuring smooth transitions.
Pros of React Navigation:
- Easy to set up and use
- Highly customizable (headers, gestures, animations)
- Strong community support and active maintenance
- Lightweight and works well with Expo
- Compatible with both Android and iOS
Cons of React Navigation:
- Performance can be a concern for complex animations (since it relies on JavaScript-based navigation rather than native components)
- Slightly slower than native navigation when rendering intricate screens
To install React Navigation, run:
npm install @react-navigation/native
npm install react-native-screens react-native-safe-area-context react-native-gesture-handler react-native-reanimated react-native-vector-icons
Then, wrap your app with a NavigationContainer
:
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
export default function App() {
return (
);
}
3. React Native Navigation (Native Navigation by Wix)
React Native Navigation by Wix is a powerful library that leverages native navigation components instead of JavaScript-based navigation, resulting in smoother performance and a truly native feel.
Pros of Native Navigation:
- Better performance and smoother animations
- Native navigation experience
- Ideal for large applications with multiple screens
- Deep linking and gesture support
Cons of Native Navigation:
- More complex setup and maintenance
- Not supported in Expo by default
- Requires linking native dependencies
To install React Native Navigation:
npm install react-native-navigation
Then, register screens in index.js
:
import { Navigation } from 'react-native-navigation';
import App from './App';
Navigation.registerComponent('HomeScreen', () => App);
Navigation.events().registerAppLaunchedListener(() => {
Navigation.setRoot({
root: {
stack: {
children: [{
component: {
name: 'HomeScreen'
}
}]
}
}
});
});
4. Comparison: React Navigation vs. Native Navigation
Feature | React Navigation | React Native Navigation |
---|---|---|
Performance | Moderate (JS-based) | High (Native-based) |
Ease of Use | Easy | More Complex |
Customization | High | Moderate |
Expo Support | Yes | No |
Animations | JS-based, limited | Native, smooth |
Best For | Small/medium apps | Large-scale apps |
5. Which One Should You Choose?
- Choose React Navigation if you need an easy-to-use, customizable, and Expo-compatible solution.
- Choose Native Navigation if your app has complex screens, heavy animations, and requires the best native performance.
Conclusion
At the end of the day, navigation is not just about getting from Point A to Point B—it’s about creating a seamless and engaging experience for your users. If you’re looking for flexibility and ease of use, React Navigation will serve you well. But if you need the absolute best performance and a truly native experience, React Native Navigation is the way to go.
Stay tuned for the next chapter of React Native Unplugged, where we’ll tackle another must-know topic to make your development process smoother!