You’ve built your React Native app, but now comes the final step—getting it into the hands of users. Publishing to the Apple App Store and Google Play Store can feel overwhelming, with all the certificates, configurations, and submission guidelines. But don’t worry! This chapter of React Native Unplugged will break it down step by step, so you can confidently launch your app to a global audience.
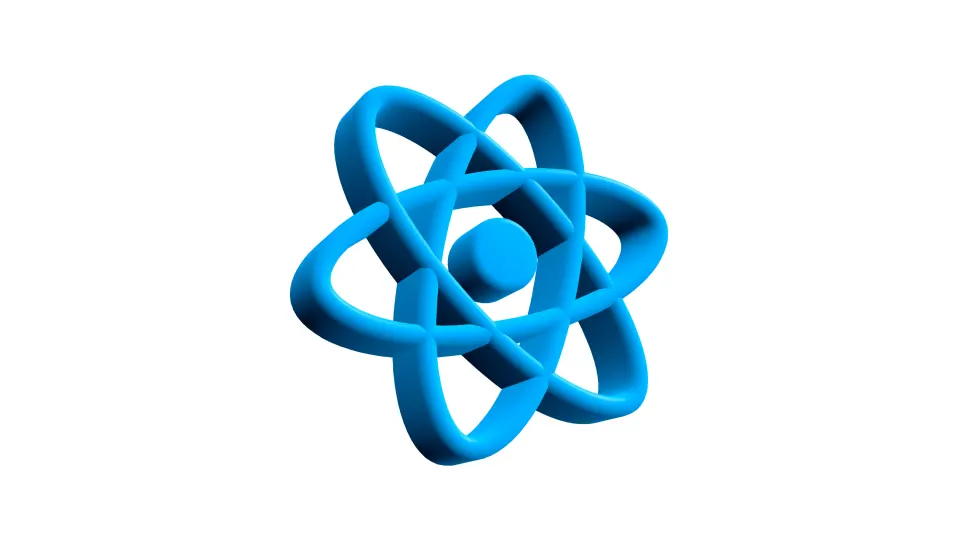
1. Preparing Your React Native App for Production
Before publishing, ensure your app is optimized and ready:
- Remove unnecessary logs (
console.log
statements). - Optimize performance (lazy loading, efficient state management).
- Test thoroughly on real devices and emulators.
- Ensure your app follows Apple and Google store guidelines.
2. Building for Android (Google Play Store)
Step 1: Generate a Keystore
A Keystore file is required to sign your app.
keytool -genkeypair -v -keystore my-release-key.jks -keyalg RSA -keysize 2048 -validity 10000 -alias my-key-alias
This command will prompt you for a password and other details. Save the generated my-release-key.jks
file in the android/app
directory.
Step 2: Configure gradle.properties
Edit android/gradle.properties
and add:
MYAPP_RELEASE_STORE_FILE=my-release-key.jks MYAPP_RELEASE_KEY_ALIAS=my-key-alias MYAPP_RELEASE_STORE_PASSWORD=your-password MYAPP_RELEASE_KEY_PASSWORD=your-password
Step 3: Configure android/app/build.gradle
Modify the signingConfigs
section:
android { signingConfigs { release { storeFile file(MYAPP_RELEASE_STORE_FILE) storePassword MYAPP_RELEASE_STORE_PASSWORD keyAlias MYAPP_RELEASE_KEY_ALIAS keyPassword MYAPP_RELEASE_KEY_PASSWORD } } buildTypes { release { signingConfig signingConfigs.release minifyEnabled true proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } }
Step 4: Build the APK or AAB
Run the following command to generate a release APK:
cd android ./gradlew assembleRelease
For AAB (Android App Bundle), which is recommended for Google Play:
./gradlew bundleRelease
Find the generated APK or AAB in android/app/build/outputs/apk/release/
.
Step 5: Upload to Google Play Store
- Go to Google Play Console
- Create a new app and fill out the details.
- Upload the
.aab
file under Production. - Complete the Store Listing, App Content, and Pricing sections.
- Submit for review!
3. Building for iOS (Apple App Store)
Step 1: Install CocoaPods (if not already installed)
cd ios pod install
Step 2: Set Up an Apple Developer Account
- Sign up at Apple Developer.
- Enroll in the Apple Developer Program ($99/year required for publishing).
Step 3: Configure Xcode for Production
- Open
ios/AppName.xcworkspace
in Xcode. - Set Bundle Identifier under Signing & Capabilities.
- Select your Team (Apple Developer account).
- Set the Deployment Target (iOS 12+ recommended).
- Enable App Store Connect Capability if needed.
Step 4: Archive & Export the App
Run the following command to build the app for release:
npx react-native run-ios --configuration Release
Alternatively, archive via Xcode:
- Open Xcode and select Product > Archive.
- Once archived, click Distribute App.
- Choose App Store Connect and follow the prompts.
Step 5: Upload the App to App Store Connect
- Open Transporter (Mac app) and upload the
.ipa
file. - Go to App Store Connect.
- Create a new app and fill out the required details.
- Set up App Store Listing, Pricing, and Privacy settings.
- Submit for review!
4. Testing Before Release
For Android:
- Test using Google Play Internal Testing before public release.
- Use Firebase Test Lab for automated testing.
For iOS:
- Use TestFlight for beta testing before publishing.
- Invite testers using App Store Connect.
5. Checklist Before Submitting
✅ Ensure all screenshots and descriptions are ready.
✅ Follow Apple and Google’s store guidelines.
✅ Test thoroughly on real devices.
✅ Enable crash reporting using Firebase Crashlytics.
✅ Monitor app performance with Flipper or App Store Analytics.
✅ Have a privacy policy URL (required by both stores).
6. Conclusion
Publishing a React Native app is the final, exciting step in your development journey. Whether you’re launching on Google Play or the App Store, following the right steps ensures a smooth submission process. By setting up the right configurations, testing on real devices, and meeting all store guidelines, you’re now ready to share your app with the world! 🚀
Now that your app is live, what’s next? Stay tuned for the next chapter of React Native Unplugged, where we’ll explore another must-know topic to elevate your development skills!