Doubt:
Why bother with const
if I can reassign with let
?
Insight: When to Use const
and let
in JavaScript for More Reliable Code
In JavaScript, let
and const
are both block-scoped, modern variable declarations introduced to replace the more error-prone var
. They serve distinct roles:
const
: This keyword creates a constant reference, so you cannot reassign the variable to a different value after its initial assignment. However, for objects and arrays, you can still modify the contents they reference becauseconst
only prevents reassignment of the reference, not changes to the object or array’s contents.let
: This keyword allows reassignment, making it useful for variables whose values will change over time (e.g., counters, accumulators, or configurations).
Example Code
Imagine Tony Stark is tracking some suit systems. Certain settings, like the suit model, should remain constant, while others, like suit power level, will need updates.
const suitModel = "Mark XLVII"; // This suit model cannot be reassigned let powerLevel = 100; // Power level will decrease over time // Attempting reassignment suitModel = "Mark L"; // Error: Assignment to constant variable powerLevel = 85; // Allowed - powerLevel can be reassigned console.log(suitModel); // Outputs: "Mark XLVII" console.log(powerLevel); // Outputs: 85
Working with const
and Objects or Arrays
It’s important to note that const
prevents reassignment of the variable, not modification of the object or array it references. Here’s an example where Tony is tracking his team members in the Avengers.
const avengers = ["Iron Man", "Thor", "Hulk"];<br>avengers.push("Black Widow"); // Allowed - modifying the array contents<br>console.log(avengers); // Outputs: ["Iron Man", "Thor", "Hulk", "Black Widow"]<br><br>// Reassigning the array itself will throw an error<br>avengers = ["Spider-Man", "Doctor Strange"]; // Error: Assignment to constant variable
In this case, const
prevents reassigning avengers
to a new array but allows operations that modify the original array’s contents, such as adding or removing items.
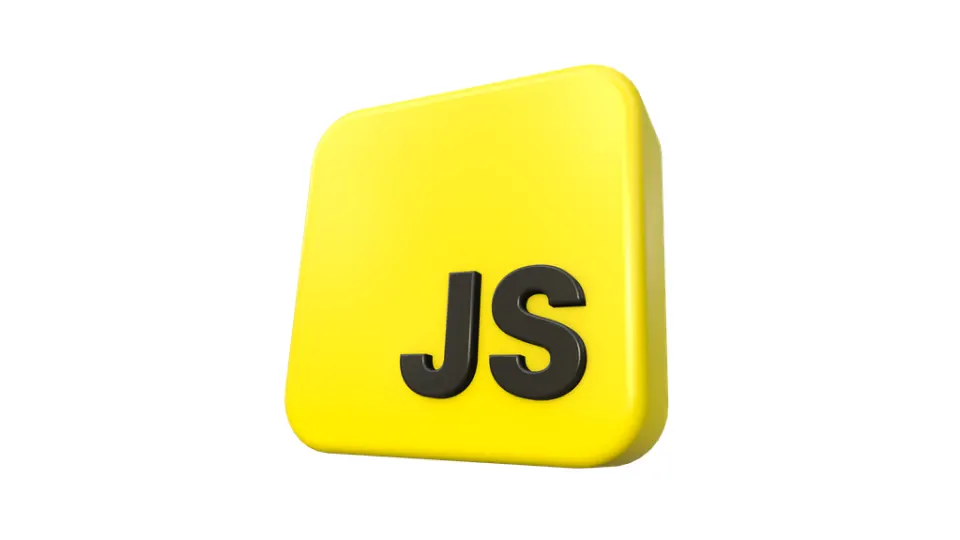
Why This Matters
- Predictability: Using
const
whenever possible creates more predictable code. You know that aconst
variable won’t change, which reduces cognitive load and helps avoid accidental bugs. - Intentional Reassignment: When a variable is declared with
let
, it signals that reassignment is intentional and likely necessary within that context, making the code more readable and intention-driven.
Best Practice: Optimal Usage Tips for let
and const
- Default to
const
for variables that shouldn’t be reassigned, and uselet
only for variables that require reassignment, such as counters or values in loops. - Switch to
let
only when reassignment is required: For example, in loops, counters, or variables that must be updated as the code progresses.
Final Takeaway: const
for Stability, let
for Flexibility
Think of const
as a safeguard that prevents unintended changes. In Tony’s world, this might be like locking down certain suit features once they’re set. By using const
as the default, you ensure that certain values remain constant, leading to more stable and predictable code. Reach for let
only when you’re working with a variable that needs flexibility.
Curious about JavaScript’s other quirks? Head over to our article on ==
vs. ===
to learn the key differences.