Doubt:
Are null
and undefined
just empty values?
Insight: Understanding null and undefined in JavaScript
In JavaScript, null
and undefined
both represent “empty” values, but they serve different purposes and are used in specific contexts. Understanding their differences can lead to cleaner, more intentional code.
undefined
: This value indicates a variable has been declared but not assigned a value, or it refers to a property that doesn’t exist on an object.
null
: This is an explicit assignment to a variable to signify the intentional absence of any value. It’s often used to indicate “no value” or “empty” when you want to reset or clear an existing value.
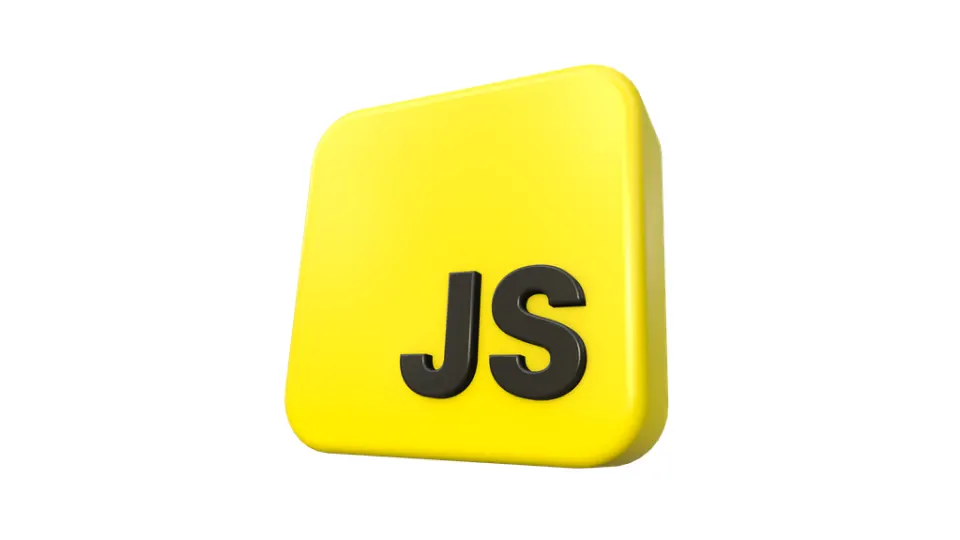
Example Code
Let’s say Tony Stark has a system for tracking his suit status and weapons, and we want to keep track of these values.
let suitStatus; // undefined - suitStatus is declared but not assigned let weapon = null; // null - weapon is explicitly set to "no value" // Undefined example - checking an uninitialized value console.log(suitStatus); // undefined - no assignment has been made // Null example - explicitly set "no value" console.log(weapon); // null - represents an intentional lack of value // Attempting to access a non-existent property let tonyProfile = { name: "Tony Stark", alias: "Iron Man" }; console.log(tonyProfile.age); // undefined - age property does not exist in the object
Why This Matters
Unintentional vs. Intentional Emptiness
undefined
represents a variable or property that is either uninitialized or doesn’t exist, usually happening unintentionally or by default.null
signifies an explicit setting of “no value.” This is useful for resetting or clearing a variable, making your intent clear to other developers.
JavaScript Behaviors with null
and undefined
undefined
is the default for unassigned variables and non-existent properties, often set automatically by JavaScript.null
is only assigned when the developer intentionally sets it.
Practical Usage
Imagine Tony has some suit systems set up, but he wants to reset certain values while others haven’t been configured yet:
let arcReactorStatus = undefined; // Not configured yet let suitAI = null; // Explicitly set to "off" or "no AI" for now console.log(arcReactorStatus); // undefined - unconfigured console.log(suitAI); // null - explicitly set to "no value" // Reassigning values arcReactorStatus = "active"; // Now the arc reactor is operational suitAI = "Jarvis"; // Now we've activated Jarvis as the suit AI
Best Practices for Using null
and undefined
in JavaScript
- Use
null
when you want to clearly signal “no value” or to reset a variable. - Rely on
undefined
for variables that are yet to be initialized or for properties that might not exist. Avoid explicitly setting a variable toundefined
, as JavaScript assignsundefined
by default in these cases.
Key Differences Between null
and undefined
undefined
: Automatically assigned by JavaScript for uninitialized variables or non-existent properties.null
: An intentional assignment to indicate “no value” or “empty.”
Final Takeaway: Writing Cleaner JavaScript with null
and undefined
While null
and undefined
may look similar, using them intentionally clarifies the code’s purpose and avoids unexpected behavior. Think of null
as Tony Stark’s “off switch” for when he’s deactivating a system, while undefined
is simply “no configuration has been applied yet.”
Curious about other JavaScript essentials? Check out our guide on JavaScript Arrays vs. Objects: The Bracket Battle to learn more about common misconceptions.