Swiper.js is a powerful JavaScript library that lets you create sleek, touch-enabled sliders with hardware-accelerated transitions. Whether you’re building a carousel, a testimonial section, or a full-screen gallery, Swiper.js has you covered. Today, I’m going to walk you through creating your very own slider in just three simple steps.
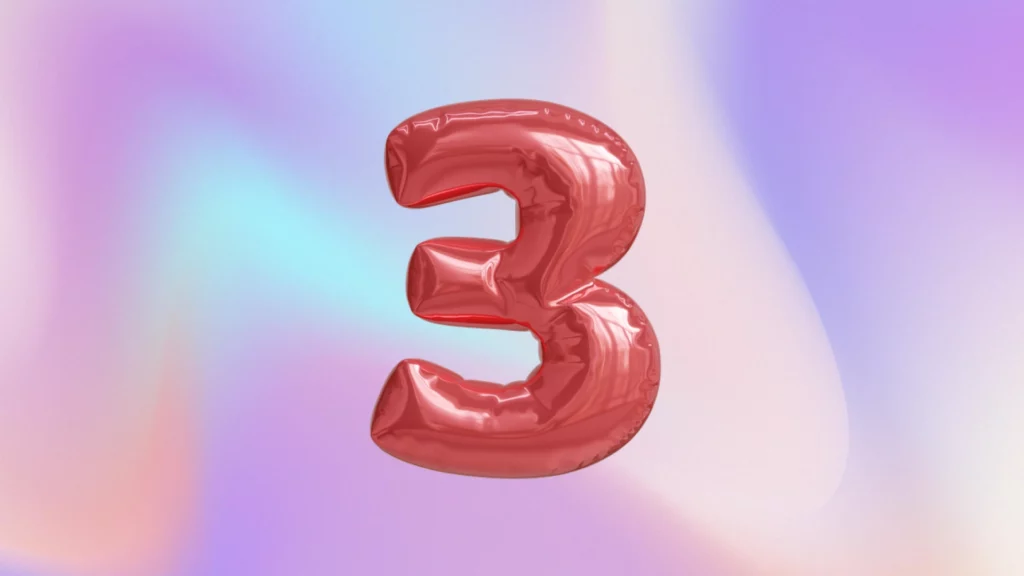
1. Import Swiper JS
Start by importing the Swiper Js library. You can install it in a few different ways—via npm, a CDN, or by directly including it in your HTML. For simplicity, we’ll go with the HTML method.
Here’s how to get it up and running:
Import the CSS file
<head>
<meta charset="utf-8" />
<title>Swiper Demo</title>
<meta name="viewport" content="width=device-width, initial-scale=1, minimum-scale=1, maximum-scale=1" />
<!-- Swiper's CSS: Required for proper functionality -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper/swiper-bundle.min.css" />
</head>
Import the JavaScript file
Place the script tag right before the closing </body>
tag to ensure it doesn’t block the rendering of the rest of your page.
<!-- Swiper JS -->
<script src="https://cdn.jsdelivr.net/npm/swiper/swiper-bundle.min.js"></script>
</body>
2. Create Your HTML Template
Next, you’ll need to set up your slider’s HTML structure. This will be the layout for your slider, including buttons for navigation and pagination.
Important: Swiper relies on specific classes to function correctly. Keep the default Swiper classes intact to avoid breaking the slider. You can, however, add custom classes if needed.
Here’s a basic HTML template for your Swiper slider:
<!-- Swiper -->
<div class="swiper bits-tips-container">
<div class="swiper-wrapper">
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
<div class="swiper-slide">Slide 4</div>
</div>
<!-- Add Pagination -->
<div class="swiper-pagination"></div>
<!-- Add Navigation Buttons -->
<div class="swiper-button-next"></div>
<div class="swiper-button-prev"></div>
</div>
3. Initialize Swiper with JavaScript
Now it’s time to bring your slider to life by initializing Swiper with some JavaScript. You can customize the Swiper object with various parameters to control the behavior of your slider, such as the slide direction, whether the slides should loop infinitely, and the elements used for navigation and pagination.
Here’s a simple initialization script:
(function () {
// Initialize Swiper:
const swiper = new Swiper(".bits-tips-container", {
direction: "horizontal", // Slide direction
loop: true, // Infinite loop
pagination: {
el: ".swiper-pagination", // Pagination element
},
navigation: {
nextEl: ".swiper-button-next", // Next button
prevEl: ".swiper-button-prev", // Previous button
},
});
})();
Conclusion
And there you have it—your very own custom slider, powered by Swiper.js! By following these three steps, you’ve created a responsive, touch-friendly slider that’s easy to customize and integrate into any project. Not only does this approach save you time, but it also enhances the user experience with smooth, hardware-accelerated transitions.
Implementing Swiper.js not only makes your site more interactive and visually appealing, but it also keeps your code clean and maintainable. Ready for more tips and tricks? Be sure to check out our other resources to level up your dev game!
✍️ This article was written by our copywriter, Sofia, in collaboration with our JS expert, Juan.