As a professional React developer, you’re no stranger to the challenges of component-based development. But even the most experienced explorers need to keep sharpening their skills and discovering new tricks to survive. So, let’s explore 10 advanced tips and tricks that will make your React apps not just functional, but exceptional in performance!
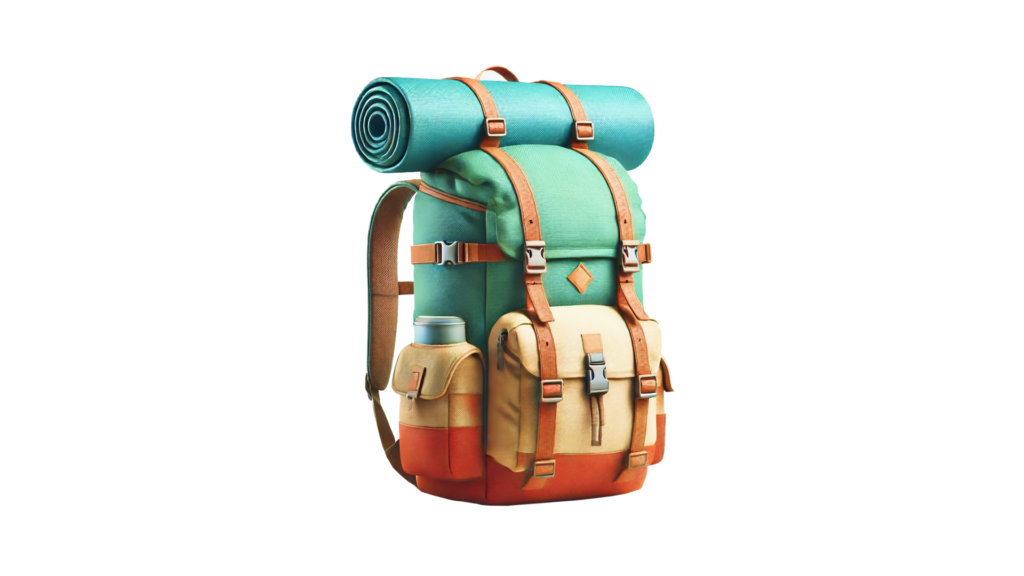
1. Memoize Expensive Functions with useMemo
In the wild, you wouldn’t want to rebuild a complex shelter every night, right? The same goes for React: use useMemo
to memoize expensive calculations so you don’t have to recalculate them on every render.
const expensiveCalculation = useMemo(() => {
return computeExpensiveValue(a, b);
}, [a, b]);
This is particularly handy when you’re dealing with large datasets or heavy computations that should only re-run when their dependencies change. It’s like setting up a semi-permanent camp—efficient and energy-saving.
2. Optimize Re-Renders with React.memo
You wouldn’t want to build the same campfire over and over again if it’s already burning bright. In React, wrap your functional components with React.memo
to prevent unnecessary re-renders when props don’t change.
const MyComponent = React.memo(({ data }) => {
return <div>{data}</div>;
});
This trick is your trusty machete, cutting down on redundant processes and keeping your app’s performance sharp.
3. Custom Hooks for Reusable Logic
Why reinvent the wheel (or the snare trap) every time? Encapsulate reusable logic in custom hooks to keep your codebase clean and modular.
function useFetch(url) {
const [data, setData] = useState(null);
useEffect(() => {
fetch(url)
.then(response => response.json())
.then(data => setData(data));
}, [url]);
return data;
}
const Component = () => {
const data = useFetch('https://api.example.com/data');
return <div>{JSON.stringify(data)}</div>;
};
This is like having a go-to kit in your backpack—easy to reach for and packed with everything you need to tackle common scenarios.
4. Avoid Prop Drilling with Context API
Deep prop drilling is the React equivalent of wandering in the jungle—it’s easy to get lost. Use React’s Context API to pass data through the component tree without prop drilling.
const UserContext = React.createContext();
const ParentComponent = () => {
return (
<UserContext.Provider value={{ name: 'John Doe' }}>
<ChildComponent />
</UserContext.Provider>
);
};
const ChildComponent = () => {
const user = useContext(UserContext);
return <div>{user.name}</div>;
};
This approach lets you cut through the complexity, making your code more maintainable and less tangled.
5. Lazy Loading Components with React.lazy
and Suspense
In survival, efficiency is key—only carry what you need, when you need it. The same applies to your React app: use React.lazy
and Suspense
to load components only when they’re needed.
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
This can significantly reduce your initial load time, helping your app feel faster and more responsive.
6. Use useCallback
to Prevent Unnecessary Function Recreation
Just as you wouldn’t carve a new spear every time you see prey, use useCallback
to memoize functions and prevent them from being recreated on every render.
const handleClick = useCallback(() => {
console.log('Button clicked!');
}, []);
return <button onClick={handleClick}>Click Me</button>;
This is important when passing functions to child components, as it prevents unnecessary re-renders and keeps your app running smoothly.
7. Leverage Error Boundaries for Robustness
In the wild, you prepare for the worst—a sudden storm, a fall, or a broken bone. Similarly, in React, use error boundaries to catch JavaScript errors in your component tree and display fallback UIs instead of crashing the entire app.
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
function App() {
return (
<ErrorBoundary>
<ComponentThatMayThrow />
</ErrorBoundary>
);
}
This ensures your app stays robust and can handle unexpected errors with grace—just like a true survivalist.
8. Use Fragments to Avoid Extra DOM Elements
You wouldn’t carry unnecessary gear, so why add extra nodes to your DOM? React Fragments lets you group multiple elements without the extra baggage.
return (
<>
<h1>Title</h1>
<p>Some content here.</p>
</>
);
This keeps your DOM clean and avoids unnecessary nesting, just like keeping your campsite tidy.
9. Optimize Rendering with Key Prop in Lists
When rendering lists, always use a unique key
prop to help React identify which items have changed.
const items = data.map(item => <li key={item.id}>{item.name}</li>);
Just as you would mark trees or leave signs to avoid getting lost in the wilderness, using unique keys helps React efficiently track changes in your lists. Proper key usage can drastically reduce re-renders and optimize performance—ensuring you never lose your way in a tangle of code.
10. Use useReducer
for Complex State Management
When the state of your app starts to resemble the complex rules of survival, switch to useReducer
. It’s more structured and predictable than juggling multiple useState
hooks.
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</>
);
}
This approach is like having a detailed survival manual—clear steps, structured logic, and a path to navigate even the trickiest scenarios.
Wrapping it Up: Use React with Advanced Techniques
These advanced tips and tricks for React are your compass in the wild. By incorporating these techniques into your projects, you’ll keep your codebase lean, your performance optimized, and your development process smooth!