Welcome back to our series on Rust web development! In this chapter, we focus on testing and debugging—key practices to ensure the quality and reliability of your web apps. We’ll explore unit testing, integration testing, and effective debugging techniques using browser dev tools with WebAssembly.
The Importance of Testing in Rust Web Apps
Testing is crucial for catching bugs early, ensuring code reliability, and maintaining a high-quality user experience. Rust’s strong type system and memory safety features already reduce common bugs, but comprehensive testing further solidifies your app’s robustness.
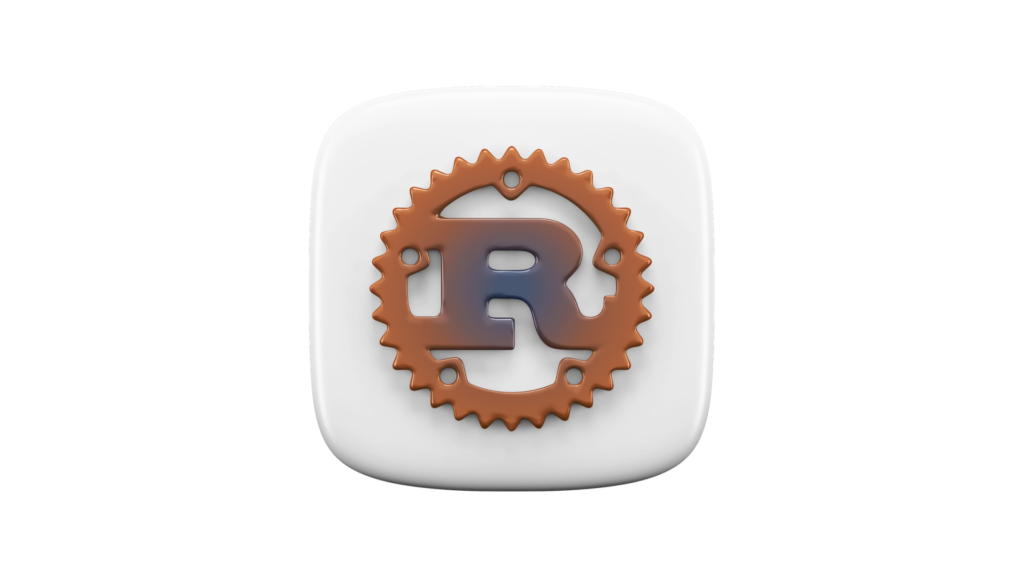
Unit Testing in Rust
Unit tests focus on individual components or functions, ensuring they work as expected in isolation. Rust’s built-in testing framework makes it straightforward to write and run unit tests.
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_addition() {
assert_eq!(2 + 2, 4);
}
}
Integration Testing in Rust
Integration tests evaluate how different parts of your application work together. These tests are placed in the tests
directory and can involve more complex scenarios than unit tests.
#[cfg(test)]
mod integration_tests {
use super::*;
#[test]
fn test_full_functionality() {
let result = complex_function(2, 3);
assert_eq!(result, 5);
}
}
Debugging with WebAssembly
When working with Rust and WebAssembly, debugging can be challenging but manageable with the right tools. Browser dev tools allow you to set breakpoints, inspect variables, and step through your WebAssembly code.
- Source Maps: Enable source maps in your build configuration to map WebAssembly code back to your original Rust source code.
- Debugging Tools: Use browser extensions or built-in dev tools to inspect and debug your WebAssembly modules.
Conclusion
Thorough testing and effective debugging are essential to developing reliable and high-quality Rust web applications. By incorporating unit and integration tests and utilizing browser dev tools for WebAssembly, you can ensure your applications are both robust and efficient. Adopting these practices will not only help you catch and fix bugs early but also maintain a seamless and enjoyable user experience.
Stay tuned for our next chapter, where we’ll guide you through the process of deploying and hosting your Rust web apps. We’ll discuss WebAssembly compatibility, server requirements, and setting up continuous integration/deployment pipelines to ensure smooth and successful deployments.