Doubt:
Why do I need ===
when ==
seems to work just fine?
Insight: Understanding Loose and Strict Equality in JavaScript
In JavaScript, understanding the difference between ==
(loose equality) and ===
(strict equality) is important for writing clear, predictable code. Here’s what’s happening under the hood:
- Loose Equality (
==
): The==
operator compares two values but allows type coercion. This means JavaScript will try to convert both values to a common type before making the comparison, sometimes leading to unexpected results where different data types appear “equal.” For example,0 == "0"
istrue
because JavaScript converts"0"
(a string) to0
(a number) before comparing. - Strict Equality (
===
): The===
operator, on the other hand, compares both value and type without any type conversion. It’s more predictable and precise, so0 === "0"
evaluates asfalse
since it respects both the type and the value.
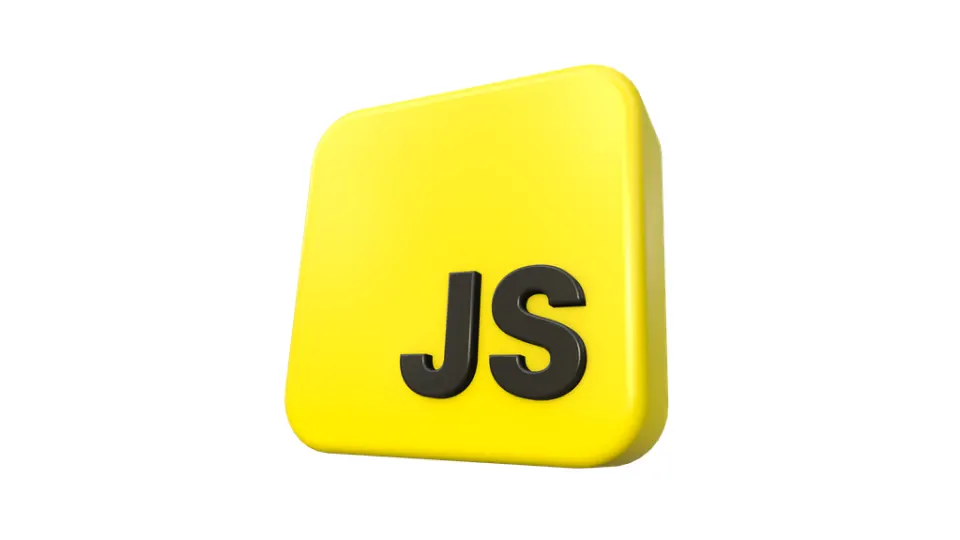
Example Code
Let’s bring in Tony Stark to see ==
and ===
in action.
Imagine Tony’s superhero profile includes attributes like his age, alias, and a status check on whether he’s in “Iron Man mode.” Here’s a small example:
let tonyAge = 48; // Number let tonyAlias = "Iron Man"; // String let ironManMode = "1"; // String, intended to represent true // Loose equality examples (==) - type coercion occurs console.log(tonyAge == "48"); // true - "48" is coerced to 48 console.log(ironManMode == true); // true - "1" is coerced to true // Strict equality examples (===) - no type coercion console.log(tonyAge === "48"); // false - types are different (number vs. string) console.log(ironManMode === true); // false - "1" (string) is not the same type as true (boolean)
Why This Matters
Type Coercion and Unexpected Results
With ==
, JavaScript’s type coercion can give results you didn’t expect, especially in complex conditions. For example, if ironManMode
is "1"
as a string, ironManMode == true
will evaluate as true
, which may seem logical but could lead to confusion when the exact data type actually matters.
Best Practice: Use ===
by Default for Predictable Comparisons
Always use ===
by default to avoid accidental coercion. This makes your comparisons more predictable and helps prevent potential bugs.
Consider ==
only when handling null
and undefined
, as null == undefined
returns true
, while null === undefined
returns false
. In cases where you’re simply checking for a value’s existence, ==
can be useful.
A Final Takeaway: Writing Cleaner JavaScript with ==
and ===
Loose equality has specific use cases, but strict equality should be your default to avoid unexpected behavior. Stick with ===
for a more reliable approach, and your code will be as dependable as Tony Stark’s armor.
Don’t stop here. For more JavaScript essentials, check out our guide on the difference between null
and undefined
to learn more.