Are you venturing into the React wilderness for the first time? It can feel like trying to build a fire in the rain—challenging, but not impossible. As Bear Grylls might say, “Improvise, adapt, overcome.” With the right know-how, you can turn those damp sticks (or confusing code snippets) into a blazing campfire of productivity. Here are three common challenges that junior React developers often face, along with some survival tips to help you tackle them head-on.
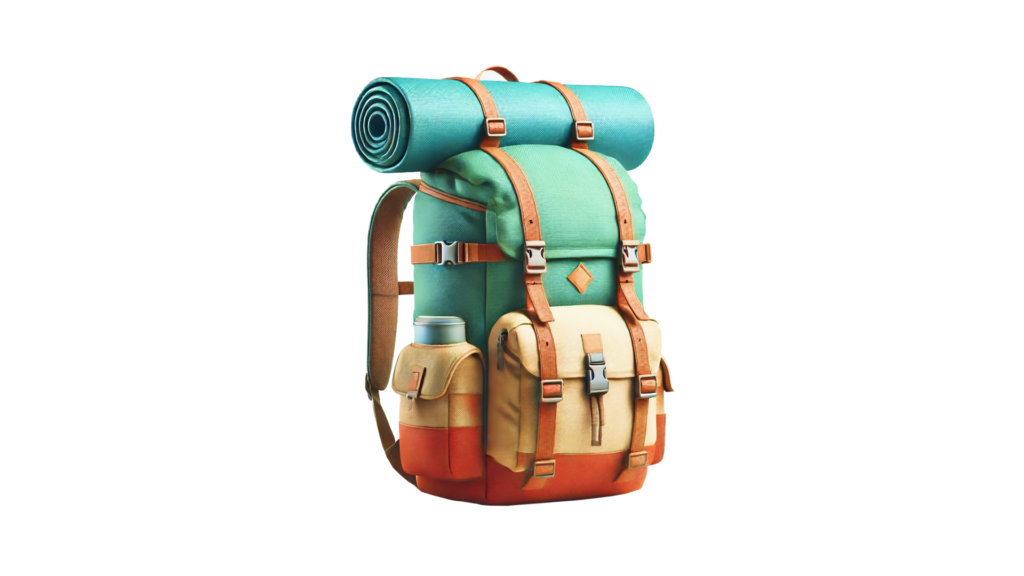
1 – State Management: The Ever-Changing Terrain
Challenge: Understanding and managing state
in React is a bit like reading a map. As your app grows, figuring out where to place state
, how to pass it around, or when to lift it up can become overwhelming. If you don’t know what you’re doing, you’ll end up going in circles, lost in a maze of your own making.
How to face it:
Start Simple with useState
Use React’s built-in useState
hook for simple components. It’s like starting with basic navigation—don’t worry about compasses or GPS until you’ve mastered the basics.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
This snippet is a simple example of how to manage state
in a functional component.
Lift State Up for Better Management
If multiple components need access to the same state, lift that state up to the nearest common ancestor and pass it down through props. It’s like climbing to higher ground for a better view—sometimes you need a broader perspective to see the path ahead.
function Parent() {
const [sharedState, setSharedState] = useState('');
return (
<>
<ChildComponent state={sharedState} setState={setSharedState} />
<AnotherChildComponent state={sharedState} />
</>
);
}
This snippet shows how to pass state
and the updater function down through props, helping manage state
across sibling components.
Explore the React Context API
When your state needs to be shared across many components, the React Context API is your friend. Think of it as your emergency radio—critical for complex situations before you venture into more advanced tools like Redux or Zustand.
import React, { useContext, useState } from 'react';
const ThemeContext = React.createContext();
function App() {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={theme}>
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar() {
const theme = useContext(ThemeContext);
return <div>Current Theme: {theme}</div>;
}
This example provides a straightforward use case for sharing state across deeply nested components without prop drilling.
Practice and Practice
Just like practicing how to start a fire, building small projects focused on state management will boost your confidence and skills. The more you practice, the more adept you’ll become at navigating the terrain.
2 – Understanding the React Lifecycle and Hooks: Timing is Everything
Challenge: React’s lifecycle methods and hooks can be as perplexing as trying to figure out when to set up camp for the night. Understanding when and how to use hooks like useEffect
, useMemo
, and useCallback
can feel like learning to read the signs of the wild.
How to face it:
Focus on useEffect
This hook is like your survival handbook—crucial for responding to changes. Learn how dependencies work and how to dodge common pitfalls, like those dreaded infinite loops that can leave you spinning in circles.
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(prev => prev + 1);
}, 1000);
return () => clearInterval(interval);
}, []);
return <div>Timer: {seconds} seconds</div>;
}
This snippet demonstrates the useEffect
hook for running side effects, like setting up an interval, and the importance of cleaning up after them.
Avoiding Common Pitfalls:
- Dependencies Matter: Ensure your dependency array is complete; otherwise, your effect might run more often than intended.
- Cleanup Functions: If your effect returns a function, React will run it to clean up before the component unmounts or before the effect re-runs. This is critical for avoiding memory leaks in things like timers, subscriptions, or event listeners.
Learn by Example and Practice
Sometimes the best way to learn is by seeing how others do it. Break down code examples and tutorials like you would a survival scenario—dissect them, understand them, and apply the knowledge to your own projects.
Utilize React Documentation
React’s official documentation is like your field guide—detailed, reliable, and always there when you need a refresher. Keep it handy and don’t hesitate to dive into it when you’re unsure of your next move.
Build Small Demos
Create mini-projects that let you focus on one hook at a time. It’s like practicing different survival skills—master each one before moving on to the next, ensuring you’re prepared for whatever challenges come your way.
3 – Handling Props and Prop Drilling: Keep Your Gear Light
Challenge: Passing data down through multiple layers of components, known as prop drilling, can become as cumbersome as lugging a heavy backpack through the jungle. If you’re not careful, you might end up with code that’s as tangled as vines.
How to face it:
Use PropTypes or TypeScript for Predictability
Learning to use PropTypes (or TypeScript) is like packing your gear with care. It ensures everything is in its place and your components are predictable and easy to debug, much like knowing exactly what’s in your backpack before setting out on a hike.
import PropTypes from 'prop-types';
function Greeting({ name, age }) {
return <p>Hello, {name}! You are {age} years old.</p>;
}
Greeting.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
This example illustrates how PropTypes
can help catch errors early by enforcing type checking on props.
Minimize Prop Drilling
Avoid deep prop drilling by designing your component hierarchy thoughtfully. Consider lifting state higher up, using component composition, or employing the React Context API. Think of it as keeping your load light—only carry what you need, and make sure your path is clear.
Understand the React Context API
When prop drilling becomes unavoidable, the React Context API is like having a supply drop—reliable and efficient, making sure your data gets where it needs to go without you breaking a sweat.
Explore TypeScript
If you’re open to it, consider using TypeScript for type-checking. It’s becoming increasingly popular in React projects, offering even stronger guarantees and improving the development experience.
Wrapping it Up: From Greenhorn to React Adventurer
Just like Bear Grylls would tell you, survival is about preparation and adaptation. By understanding state management, mastering the React lifecycle and hooks, and efficiently handling props, junior developers can transform from greenhorns to seasoned React adventurers.
Keep practicing, stay curious, and remember: every challenge is just another opportunity to sharpen your survival skills!