Doubt:
What’s the big deal with Promises and async/await
? Why not stick with callbacks?
Insight: A Guide to Smoother Asynchronous Code in JavaScript
JavaScript is single-threaded, meaning it can only process one task at a time on the main thread. However, asynchronous operations, like fetching data or setting a timeout, do not block the main thread. Instead, these operations are managed by the browser’s Web APIs (or Node.js’s environment), which handle them in the background.
Once these tasks are complete, they are added back to JavaScript’s main thread via the event loop. This mechanism allows JavaScript to continue executing other code while it waits for asynchronous operations to finish, making the language non-blocking and responsive.
Here’s the evolution of asynchronous handling in JavaScript:
- Callbacks: The original way to handle async tasks, but they can lead to “callback hell” when you have multiple nested callbacks. This structure makes code harder to read, debug, and maintain.
- Promises: Introduced as a cleaner alternative to callbacks, Promises make it easier to handle async tasks with methods like
.then()
for chaining and.catch()
for error handling. But with extensive chaining, Promises can still get verbose. async/await
: Built on Promises,async/await
adds syntactic sugar that makes async code look and behave more like synchronous code. Withtry/catch
blocks for error handling,async/await
improves readability and simplifies complex async flows.
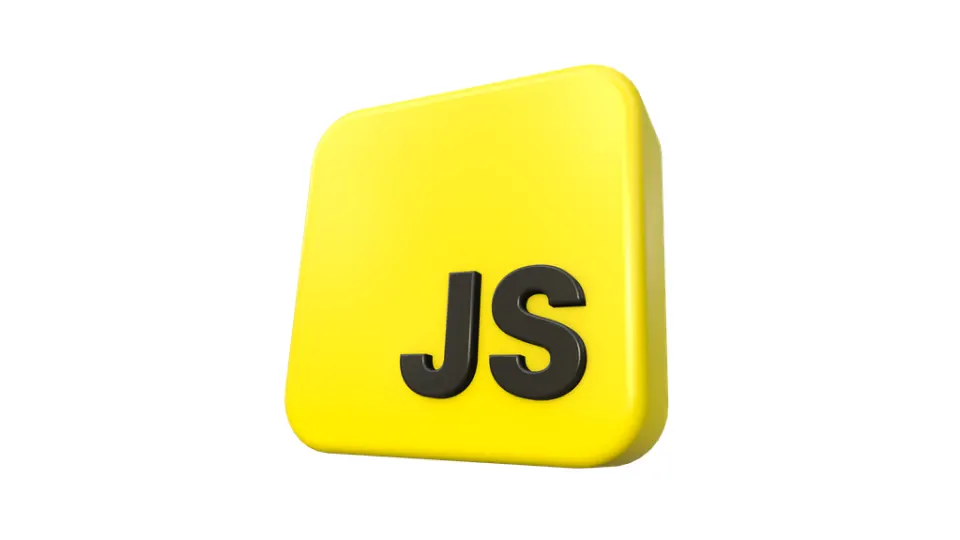
Example Code
Let’s say Tony Stark wants to retrieve his suit status, recharge it if needed, and then report the final status. We’ll compare the callback approach to the Promise and async/await
approaches.
Callback Approach: Simple but Risky for Readability
Here’s what it looks like to nest callbacks, also known as “callback hell.”
function getSuitStatus(callback) { setTimeout(() => { console.log("Retrieved suit status."); callback(); }, 1000); } function rechargeSuit(callback) { setTimeout(() => { console.log("Suit recharged."); callback(); }, 1000); } function reportStatus() { console.log("Suit status is fully operational."); } // Nested callbacks getSuitStatus(() => { rechargeSuit(() => { reportStatus(); }); });
While this works, it quickly becomes hard to read as more nested callbacks are added. Also, error handling requires each function to handle errors individually, leading to inconsistent or scattered error management.
The Promise Approach: Improving Syntax and Error Handling
With Promises, we get a cleaner, more readable syntax with chaining.
function getSuitStatus() { return new Promise((resolve) => { setTimeout(() => { console.log("Retrieved suit status."); resolve(); }, 1000); }); } function rechargeSuit() { return new Promise((resolve) => { setTimeout(() => { console.log("Suit recharged."); resolve(); }, 1000); }); } // Chaining Promises getSuitStatus() .then(() => rechargeSuit()) .then(() => console.log("Suit status is fully operational.")) .catch((error) => console.error("Error:", error));
With Promises, we can handle errors in one place using .catch()
. This is already more readable than the nested callback approach, but with complex chains, the code can still become lengthy.
async/await: A Clean, Readable Solution for Asynchronous Code
async/await
provides the cleanest, most readable syntax by making asynchronous code look synchronous. It also allows us to use try/catch
for centralized error handling.
async function handleSuitStatus() { try { await getSuitStatus(); await rechargeSuit(); console.log("Suit status is fully operational."); } catch (error) { console.error("Error:", error); } } handleSuitStatus();
With async/await
, each asynchronous call is preceded by await
, making the code flow from top to bottom in a way that’s easier to read and maintain. Error handling is consolidated with try/catch
, making debugging and error handling much simpler.
Why async/await Matters for Clean JavaScript Code
- Readability:
async/await
makes asynchronous code more readable, as it follows a top-to-bottom flow that’s similar to synchronous code. - Centralized Error Handling: With
try/catch
inasync/await
, error handling is more consistent and easier to manage than scattered.catch()
methods or callback-based error handling. - Avoiding Callback Hell: By eliminating deeply nested functions,
async/await
simplifies complex workflows, especially as the number of async operations grows.
Best Practices for Using Callbacks, Promises, and async/await
- Use
async/await
whenever possible for handling async code. This will improve code readability and maintainability. - Reserve Promises for cases where you need Promise-specific methods like
Promise.all()
orPromise.race()
. - Use Callbacks only when necessary, such as in event-driven patterns or when working with APIs that don’t support Promises. In cases like handling a button click or a file read event, callbacks can still be effective.
Final Thoughts: Choosing the Right Asynchronous Tool
While callbacks have their place, they can lead to complex and hard-to-maintain code when managing multiple async operations. Promises and async/await
provide modern alternatives that improve readability, simplify error handling, and reduce the risk of “callback hell.” For Tony Stark, this approach might be like upgrading from manual suit controls to a high-tech AI system that handles everything smoothly.
Keep your JavaScript skills sharp! Check out our comparison of let
and const
to learn when and why it matters.